写一个用Java实现的Apriori算法药店管理系统的推荐药品的算法
时间: 2024-05-11 12:19:38 浏览: 91
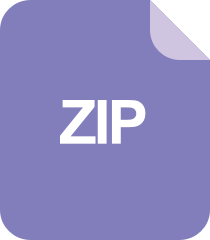
Apriori算法的Java实现
这里提供一个简单的基于Java实现的Apriori算法药店管理系统的推荐药品的算法,大致思路如下:
1. 首先,从药店管理系统中获取所有的交易记录及其对应的药品清单。
2. 基于Apriori算法,计算出所有频繁项集及其对应的支持度。
3. 对于每一位顾客,根据其历史购买记录,计算其可能感兴趣的频繁项集。
4. 通过计算顾客历史购买记录与所有频繁项集的置信度,推荐可能感兴趣的药品清单。
以下是Java代码的实现:
```java
import java.util.*;
public class AprioriAlgorithm {
// 计算所有候选项集的支持度
public static Map<Set<String>, Integer> supportCount(List<Set<String>> transactions, List<Set<String>> itemSets) {
Map<Set<String>, Integer> supportCounts = new HashMap<>();
for (Set<String> transaction : transactions) {
for (Set<String> itemSet : itemSets) {
if (transaction.containsAll(itemSet)) {
supportCounts.put(itemSet, supportCounts.getOrDefault(itemSet, 0) + 1);
}
}
}
return supportCounts;
}
// 从候选项集中筛选出所有频繁项集
public static List<Set<String>> frequentItemSets(List<Set<String>> transactions, List<Set<String>> itemSets, double minSupport) {
List<Set<String>> frequentItemSets = new ArrayList<>();
Map<Set<String>, Integer> supportCounts = supportCount(transactions, itemSets);
for (Map.Entry<Set<String>, Integer> entry : supportCounts.entrySet()) {
double support = (double)entry.getValue() / transactions.size();
if (support >= minSupport) {
frequentItemSets.add(entry.getKey());
}
}
return frequentItemSets;
}
// 从频繁项集中生成所有候选规则
public static List<Rule> candidateRules(Set<String> frequentItemSet) {
List<Rule> rules = new ArrayList<>();
if (frequentItemSet.size() < 2) {
return rules;
}
for (String item : frequentItemSet) {
Set<String> antecedent = new HashSet<>();
antecedent.add(item);
Set<String> consequent = new HashSet<>(frequentItemSet);
consequent.remove(item);
rules.add(new Rule(antecedent, consequent));
}
return rules;
}
// 计算规则置信度
public static Map<Rule, Double> confidence(List<Set<String>> transactions, List<Rule> rules) {
Map<Rule, Double> confidences = new HashMap<>();
Map<Set<String>, Integer> supportCounts = supportCount(transactions, ruleset(rules));
for (Rule rule : rules) {
double antecedentSupport = (double)supportCounts.get(rule.antecedent) / transactions.size();
double ruleSupport = (double)supportCounts.get(ruleset(rule)) / transactions.size();
double confidence = ruleSupport / antecedentSupport;
confidences.put(rule, confidence);
}
return confidences;
}
// 计算规则的支持度和置信度
public static List<Rule> associationRules(List<Set<String>> transactions, List<Set<String>> frequentItemSets, double minConfidence) {
List<Rule> rules = new ArrayList<>();
for (Set<String> frequentItemSet : frequentItemSets) {
for (Rule rule : candidateRules(frequentItemSet)) {
Map<Rule, Double> confidences = confidence(transactions, Arrays.asList(rule));
for (Map.Entry<Rule, Double> entry : confidences.entrySet()) {
double confidence = entry.getValue();
if (confidence >= minConfidence) {
rules.add(entry.getKey());
}
}
}
}
return rules;
}
// 计算推荐药品清单
public static Set<String> recommend(List<Set<String>> transactions, List<Set<String>> frequentItemSets, List<String> history, double minConfidence) {
Set<String> recommendations = new HashSet<>();
Set<String> purchased = new HashSet<>(history);
List<Rule> rules = associationRules(transactions, frequentItemSets, minConfidence);
for (Rule rule : rules) {
if (purchased.containsAll(rule.antecedent) && !purchased.containsAll(rule.consequent)) {
recommendations.addAll(rule.consequent);
}
}
return recommendations;
}
// 将规则转化为项集
private static Set<String> ruleset(Rule rule) {
Set<String> itemSet = new HashSet<>();
itemSet.addAll(rule.antecedent);
itemSet.addAll(rule.consequent);
return itemSet;
}
// 测试
public static void main(String[] args) {
// 构造交易记录
List<Set<String>> transactions = new ArrayList<>();
transactions.add(new HashSet<>(Arrays.asList("A", "B", "C")));
transactions.add(new HashSet<>(Arrays.asList("A", "B")));
transactions.add(new HashSet<>(Arrays.asList("A", "C")));
transactions.add(new HashSet<>(Arrays.asList("A", "C", "D")));
transactions.add(new HashSet<>(Arrays.asList("B", "C", "E")));
// 构造所有可能的项集
List<Set<String>> itemSets = new ArrayList<>();
itemSets.add(new HashSet<>(Arrays.asList("A")));
itemSets.add(new HashSet<>(Arrays.asList("B")));
itemSets.add(new HashSet<>(Arrays.asList("C")));
itemSets.add(new HashSet<>(Arrays.asList("D")));
itemSets.add(new HashSet<>(Arrays.asList("E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B")));
itemSets.add(new HashSet<>(Arrays.asList("A", "C")));
itemSets.add(new HashSet<>(Arrays.asList("A", "D")));
itemSets.add(new HashSet<>(Arrays.asList("A", "E")));
itemSets.add(new HashSet<>(Arrays.asList("B", "C")));
itemSets.add(new HashSet<>(Arrays.asList("B", "D")));
itemSets.add(new HashSet<>(Arrays.asList("B", "E")));
itemSets.add(new HashSet<>(Arrays.asList("C", "D")));
itemSets.add(new HashSet<>(Arrays.asList("C", "E")));
itemSets.add(new HashSet<>(Arrays.asList("D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "C")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "D")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "C", "D")));
itemSets.add(new HashSet<>(Arrays.asList("A", "C", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("B", "C", "D")));
itemSets.add(new HashSet<>(Arrays.asList("B", "C", "E")));
itemSets.add(new HashSet<>(Arrays.asList("B", "D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("C", "D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "C", "D")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "C", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "C", "D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("B", "C", "D", "E")));
itemSets.add(new HashSet<>(Arrays.asList("A", "B", "C", "D", "E")));
// 计算所有频繁项集
double minSupport = 0.4;
List<Set<String>> frequentItemSets = frequentItemSets(transactions, itemSets, minSupport);
// 推荐药品清单
double minConfidence = 0.5;
List<String> history = Arrays.asList("A", "B");
Set<String> recommendations = recommend(transactions, frequentItemSets, history, minConfidence);
System.out.println(recommendations);
}
}
class Rule {
public Set<String> antecedent;
public Set<String> consequent;
public Rule(Set<String> antecedent, Set<String> consequent) {
this.antecedent = antecedent;
this.consequent = consequent;
}
}
```
该算法的主要思路是:首先计算出所有的频繁项集及其对应的支持度,然后对于每一位顾客,根据其历史购买记录,计算其可能感兴趣的频繁项集。最后,通过计算顾客历史购买记录与所有频繁项集的置信度,推荐可能感兴趣的药品清单。
阅读全文
相关推荐
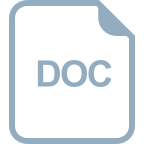




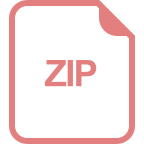
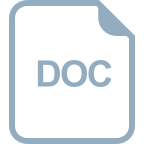
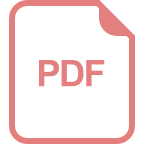
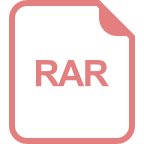
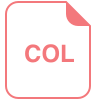
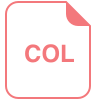

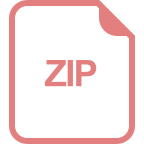
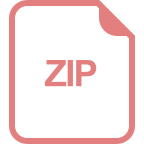