为UNet网络加入稀疏表示模块的代码
时间: 2024-04-07 21:30:33 浏览: 24
以下是一个简单的示例代码,展示了如何为UNet网络加入稀疏表示模块。请注意,这只是一个示例,具体实现可能需要根据你的数据和网络结构进行适当调整。
```python
import torch
import torch.nn as nn
# 定义UNet网络的编码器部分
class Encoder(nn.Module):
def __init__(self):
super(Encoder, self).__init__()
# 添加稀疏表示模块
self.sparse_module = SparseModule()
# 编码器的其他层
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, padding=1)
self.relu1 = nn.ReLU(inplace=True)
# ...
def forward(self, x):
# 使用稀疏表示模块选择性地激活一部分通道或特征
x = self.sparse_module(x)
# 编码器的其他层的前向传播
x = self.conv1(x)
x = self.relu1(x)
# ...
return x
# 定义UNet网络的解码器部分
class Decoder(nn.Module):
def __init__(self):
super(Decoder, self).__init__()
# 添加稀疏表示模块
self.sparse_module = SparseModule()
# 解码器的其他层
# ...
def forward(self, x):
# 使用稀疏表示模块选择性地激活一部分通道或特征
x = self.sparse_module(x)
# 解码器的其他层的前向传播
# ...
return x
# 定义稀疏表示模块
class SparseModule(nn.Module):
def __init__(self):
super(SparseModule, self).__init__()
# 在这里定义稀疏表示模块的具体操作,可以使用门控机制或注意力机制来实现
def forward(self, x):
# 在这里实现稀疏表示模块的前向传播操作
return x
# 定义完整的UNet网络
class UNet(nn.Module):
def __init__(self):
super(UNet, self).__init__()
self.encoder = Encoder()
self.decoder = Decoder()
def forward(self, x):
x = self.encoder(x)
x = self.decoder(x)
return x
# 创建UNet网络实例并进行训练
model = UNet()
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# 进行训练过程
for epoch in range(num_epochs):
# 前向传播
outputs = model(inputs)
loss = criterion(outputs, targets)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印损失等训练信息
if (epoch+1) % 10 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
```
请注意,上述代码仅为示例,实际使用时需要根据具体情况进行调整,例如定义稀疏表示模块的具体操作和参数。同时,还需要根据你的数据和任务来设置适当的损失函数、优化器和训练过程。
相关推荐
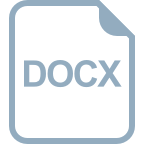














