python实现面部表情检测的代码
时间: 2023-09-04 17:11:26 浏览: 122
可以使用OpenCV和深度学习模型实现面部表情检测。以下是一个基本的示例代码:
首先需要安装必要的库:
```python
pip install opencv-python
pip install tensorflow
pip install keras
```
然后可以使用FER2013数据集训练一个简单的模型:
```python
import numpy as np
import pandas as pd
from keras.utils import np_utils
from keras.models import Sequential
from keras.layers import Dense, Dropout, Activation, Flatten
from keras.layers.convolutional import Conv2D, MaxPooling2D
from keras.optimizers import Adam
from keras.preprocessing.image import ImageDataGenerator
# 加载数据
data = pd.read_csv('fer2013.csv')
# 处理数据
pixels = data['pixels'].tolist()
faces = []
for pixel_sequence in pixels:
face = [int(pixel) for pixel in pixel_sequence.split(' ')]
face = np.asarray(face).reshape(48, 48)
faces.append(face.astype('float32'))
faces = np.asarray(faces)
faces = np.expand_dims(faces, -1)
emotions = pd.get_dummies(data['emotion']).as_matrix()
# 数据集划分
x_train, y_train = faces[:28709], emotions[:28709]
x_test, y_test = faces[28709:], emotions[28709:]
# 构建模型
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(48, 48, 1)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Conv2D(128, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(128, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(1024, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(7, activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer=Adam(lr=0.0001, decay=1e-6), metrics=['accuracy'])
# 数据增强
gen = ImageDataGenerator(rotation_range=8, width_shift_range=0.08, shear_range=0.3,
height_shift_range=0.08, zoom_range=0.08)
test_gen = ImageDataGenerator()
train_generator = gen.flow(x_train, y_train, batch_size=64)
test_generator = test_gen.flow(x_test, y_test, batch_size=64)
# 训练模型
model.fit_generator(train_generator, steps_per_epoch=28709 // 64, epochs=50,
validation_data=test_generator, validation_steps=7178 // 64)
```
训练完成后,可以使用模型进行预测:
```python
import cv2
# 加载模型
model = keras.models.load_model('model.h5')
# 加载分类标签
emotions = ['Angry', 'Disgust', 'Fear', 'Happy', 'Sad', 'Surprise', 'Neutral']
# 加载图像
img = cv2.imread('test.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 裁剪出人脸
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
faces = face_cascade.detectMultiScale(gray, 1.3, 5)
# 预测表情
for (x, y, w, h) in faces:
face = gray[y:y+h, x:x+w]
face = cv2.resize(face, (48, 48))
face = face.astype('float32') / 255.0
face = np.expand_dims(face, -1)
face = np.expand_dims(face, 0)
emotion = model.predict(face)[0]
label = emotions[np.argmax(emotion)]
cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.putText(img, label, (x, y), cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 0, 0), 2)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码会加载之前训练好的模型,并在一张测试图片上进行预测和可视化。
阅读全文
相关推荐
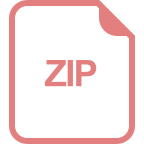
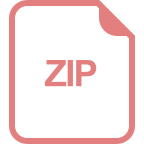
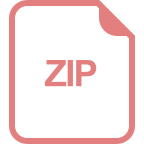
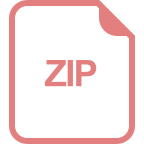

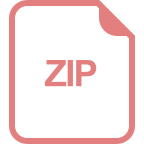
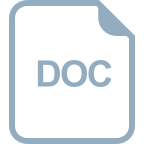
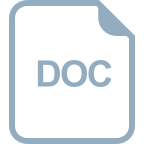
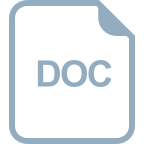
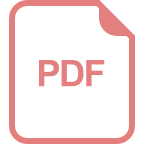
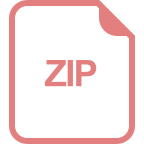
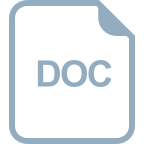
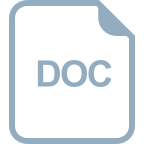
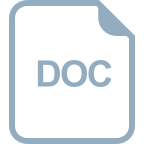
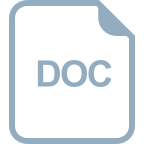
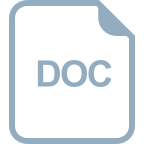

