设计一个Java程序,完成: 生成10个复数对象,通过对象序列化方式,写入到文件complex.ser文件中。然后再从该complex.ser中读入这10个复数对象,并在屏幕中输出这些对象。
时间: 2024-03-23 08:37:19 浏览: 115
好的,下面是Java程序的实现:
```java
import java.io.*;
class Complex implements Serializable {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public String toString() {
if (imag >= 0) {
return real + "+" + imag + "i";
} else {
return real + "-" + (-imag) + "i";
}
}
}
public class ComplexSerializationDemo {
public static void main(String[] args) {
// 生成10个复数对象
Complex[] arr = new Complex[10];
for (int i = 0; i < 10; i++) {
arr[i] = new Complex(Math.random(), Math.random());
}
// 序列化并写入文件
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("complex.ser"))) {
oos.writeObject(arr);
System.out.println("序列化完成");
} catch (IOException e) {
e.printStackTrace();
}
// 从文件中读取并反序列化
Complex[] arr2 = null;
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream("complex.ser"))) {
arr2 = (Complex[]) ois.readObject();
System.out.println("反序列化完成");
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
// 输出反序列化结果
if (arr2 != null) {
for (Complex c : arr2) {
System.out.println(c);
}
}
}
}
```
程序首先定义了一个复数类 `Complex`,实现了 `Serializable` 接口。然后在 `main` 方法中,生成了10个随机复数对象,并将它们序列化并写入文件 `complex.ser` 中。接着从文件中读取并反序列化,最后输出反序列化结果。
注意,程序中使用了 `try-with-resources` 语句来自动关闭输入输出流。同时,序列化和反序列化的对象类型必须一致,因此在反序列化时需要将读取到的对象转换为 `Complex` 类型。
阅读全文
相关推荐
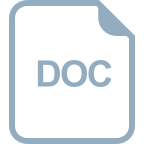
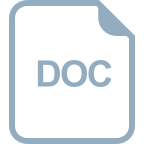
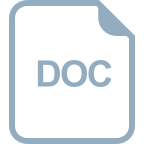









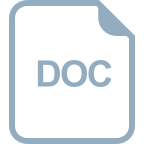
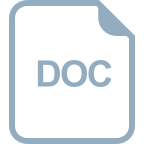
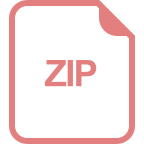
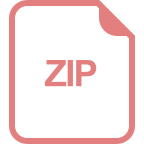
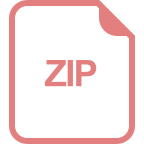