设计一个Java程序,完成: 生成10个复数对象(复数类的设计在前面作业中已做过),通过对象序列化方式,写入到文件complex.ser文件中。然后再从该complex.ser中读入这10个复数对象,并在屏幕中输出这些对象。
时间: 2024-03-24 22:41:22 浏览: 47
好的,这是一个简单的Java程序,它可以生成10个复数对象,并将它们序列化到文件中,然后再从文件中读取这些对象并输出到屏幕上。
```java
import java.io.*;
public class ComplexSerialization {
public static void main(String[] args) {
// 生成10个复数对象
Complex[] complexArray = new Complex[10];
for (int i = 0; i < 10; i++) {
complexArray[i] = new Complex(i+1, i+2);
}
// 序列化到文件
try {
FileOutputStream fileOut = new FileOutputStream("complex.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(complexArray);
out.close();
fileOut.close();
System.out.println("Serialized data is saved in complex.ser");
} catch (IOException i) {
i.printStackTrace();
}
// 从文件中读取并输出到屏幕上
try {
FileInputStream fileIn = new FileInputStream("complex.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Complex[] newComplexArray = (Complex[]) in.readObject();
in.close();
fileIn.close();
for (Complex c : newComplexArray) {
System.out.println(c);
}
} catch (IOException i) {
i.printStackTrace();
return;
} catch (ClassNotFoundException c) {
System.out.println("Complex class not found");
c.printStackTrace();
return;
}
}
}
// 复数类
class Complex implements Serializable {
private static final long serialVersionUID = 1L;
private double real;
private double imaginary;
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public String toString() {
return real + " + " + imaginary + "i";
}
}
```
这个程序会生成10个复数对象,然后将它们序列化到文件`complex.ser`中。接着,它会从`complex.ser`中读取这些对象,并将它们输出到屏幕上。请注意,`Complex`类必须实现`Serializable`接口,以便将对象序列化和反序列化。
阅读全文
相关推荐
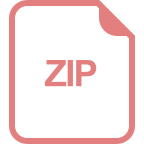
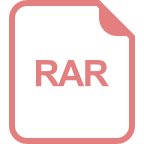
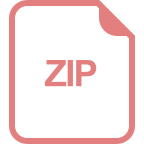















