Python写车牌识别代码
时间: 2023-11-19 11:21:41 浏览: 133
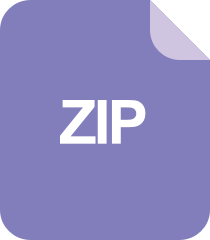
基于python下的 车牌识别代码
很高兴回答你的问题!我了解一些与Python写车牌识别相关的知识,可以和你分享一些建议。你可以使用Python开发基于图像处理的车牌识别系统,这个系统可以使用OpenCV这样的库来处理图像。你需要使用图像预处理技术来定位车牌的位置,然后使用字符分割算法将车牌上的字符分离出来,最后使用字符识别技术将字符识别出来并输出车牌号码。希望这个答案对你有用!
阅读全文
相关推荐
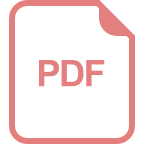

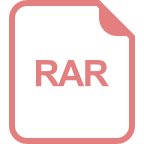
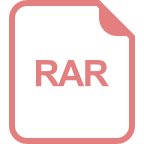












