用Verilog写一个以0xFF为头包,波特率为9600,接收4个字节,然后再以相同的顺序发送,先发0x55,数据放在IO口上的代码
时间: 2023-05-25 19:04:31 浏览: 59
以下是一个基本的Verilog代码,用于实现以0xFF为头包,波特率为9600,接收并发送四个字节的操作:
```
module uart_tx_rx(
input clk,
input rstn,
input rx,
output reg tx,
inout [7:0] io_data
);
reg [2:0] bit_count;
reg [7:0] rx_data;
reg rx_start;
reg rx_done;
reg [1:0] tx_state;
reg [7:0] tx_data;
reg [4:0] tx_bit_count;
reg tx_done;
// RX
always @(posedge clk) begin
if (!rstn) begin
bit_count <= 0;
rx_data <= 8'h00;
rx_start <= 0;
rx_done <= 0;
end
else if (rx_start) begin
if (bit_count < 8) begin
rx_data[bit_count] <= rx;
bit_count <= bit_count + 1;
end
else begin
rx_done <= 1;
rx_start <= 0;
bit_count <= 0;
end
end
else if (rx && !rx_done && !rx_start) begin
rx_start <= 1;
end
end
// TX
always @(posedge clk) begin
if (!rstn) begin
tx_state <= 0;
end
else begin
case(tx_state)
0: begin
if (rx_done && (rx_data == 8'hFF)) begin // 接收头包
tx_state <= 1;
tx_data <= 8'h55; // 发送0x55
tx_done <= 0;
tx_bit_count <= 0;
end
end
1: begin
if (tx_bit_count < 8) begin
tx <= tx_data[tx_bit_count];
tx_bit_count <= tx_bit_count + 1;
end
else begin
tx_bit_count <= 0;
tx_state <= 2;
end
end
2: begin
if (tx_bit_count < 8) begin
tx <= io_data[tx_bit_count];
tx_bit_count <= tx_bit_count + 1;
end
else begin
tx_bit_count <= 0;
tx_state <= 3;
end
end
3: begin
if (tx_bit_count < 8) begin
tx <= io_data[tx_bit_count];
tx_bit_count <= tx_bit_count + 1;
end
else begin
tx_bit_count <= 0;
tx_state <= 4;
end
end
4: begin
if (tx_bit_count < 8) begin
tx <= io_data[tx_bit_count];
tx_bit_count <= tx_bit_count + 1;
end
else begin
tx_bit_count <= 0;
tx_state <= 5;
end
end
5: begin
if (tx_bit_count < 8) begin
tx <= io_data[tx_bit_count];
tx_bit_count <= tx_bit_count + 1;
end
else begin
tx_done <= 1;
tx_state <= 6;
end
end
6: begin
if (tx_done) begin
tx_state <= 0;
end
end
endcase
end
end
endmodule
```
该代码使用了两个状态机:一个用于接收四个字节的数据包,另一个用于发送接收到的数据包。其中,发送状态机的第一个状态用于检测头包。如果接收头包,发送状态机将进入第二个状态,发送0x55。然后,它将进入三个连续的状态,将接收到的四个字节写入IO口。最后,它将进入一个完成状态,并在下一个时钟周期中返回状态机的起始状态。
请注意,这是一个基本版本的代码,实际上可能需要进行一些更改以实现特定的应用程序要求(例如,数据包结构可能需要更改或波特率可能需要更改)。
相关推荐
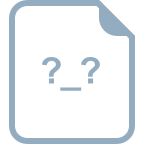
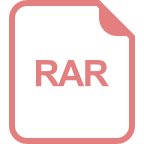














