编写Java代码,实现hive UDTF函数,函数需要传入两个String类型参数
时间: 2024-03-06 19:46:49 浏览: 67
好的,下面是一个简单的Java代码,实现了一个hive UDTF函数,函数名为`my_udtf`,需要传入两个String类型参数`str1`和`str2`:
```java
import org.apache.hadoop.hive.ql.exec.Description;
import org.apache.hadoop.hive.ql.exec.UDFArgumentException;
import org.apache.hadoop.hive.ql.exec.UDFArgumentLengthException;
import org.apache.hadoop.hive.ql.exec.UDFType;
import org.apache.hadoop.hive.ql.udf.generic.GenericUDTF;
import org.apache.hadoop.hive.serde2.objectinspector.ObjectInspector;
import org.apache.hadoop.hive.serde2.objectinspector.primitive.PrimitiveObjectInspectorFactory;
import org.apache.hadoop.hive.serde2.objectinspector.ObjectInspectorFactory;
import org.apache.hadoop.hive.serde2.objectinspector.StructObjectInspector;
import org.apache.hadoop.hive.serde2.objectinspector.primitive.PrimitiveObjectInspector;
import org.apache.hadoop.io.Text;
import java.util.ArrayList;
@Description(name = "my_udtf", value = "_FUNC_(str1, str2) - returns two strings as columns")
@UDFType(deterministic = true, stateful = false)
public class MyUDTF extends GenericUDTF {
private PrimitiveObjectInspector stringOI = null;
@Override
public StructObjectInspector initialize(ObjectInspector[] args) throws UDFArgumentException {
if (args.length != 2) {
throw new UDFArgumentLengthException("my_udtf only takes two arguments");
}
if (args[0].getCategory() != ObjectInspector.Category.PRIMITIVE
|| ((PrimitiveObjectInspector) args[0]).getPrimitiveCategory() != PrimitiveObjectInspector.PrimitiveCategory.STRING) {
throw new UDFArgumentException("my_udtf first argument must be a string");
}
if (args[1].getCategory() != ObjectInspector.Category.PRIMITIVE
|| ((PrimitiveObjectInspector) args[1]).getPrimitiveCategory() != PrimitiveObjectInspector.PrimitiveCategory.STRING) {
throw new UDFArgumentException("my_udtf second argument must be a string");
}
stringOI = (PrimitiveObjectInspector) args[0];
ArrayList<String> fieldNames = new ArrayList<String>();
ArrayList<ObjectInspector> fieldOIs = new ArrayList<ObjectInspector>();
fieldNames.add("col1");
fieldNames.add("col2");
fieldOIs.add(PrimitiveObjectInspectorFactory.writableStringObjectInspector);
fieldOIs.add(PrimitiveObjectInspectorFactory.writableStringObjectInspector);
return ObjectInspectorFactory.getStandardStructObjectInspector(fieldNames, fieldOIs);
}
@Override
public void process(Object[] record) throws UDFArgumentException {
String str1 = (String) stringOI.getPrimitiveJavaObject(record[0]);
String str2 = (String) stringOI.getPrimitiveJavaObject(record[1]);
forward(new Text(str1), new Text(str2));
}
@Override
public void close() throws UDFArgumentException {
}
}
```
这个函数会返回一个包含两个字符串列的结果集,可以在hive中使用类似以下的语句调用:
```sql
SELECT my_udtf('hello', 'world') FROM my_table;
```
其中`my_table`是你的hive表名。
阅读全文
相关推荐
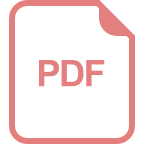

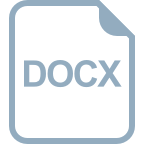
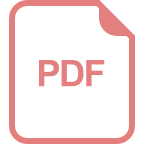
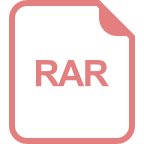
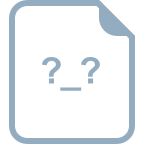
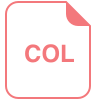
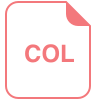
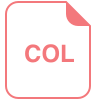
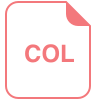





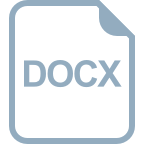
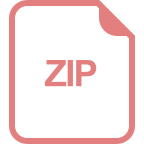