请用c++写一段qt程序,读取图片/home/nvidia/a.jpg,通过tcp传输发送至另一台主机,本地IP为192.168.1.131,目的IP为192.168.1.201,端口为22222,要求写出收发两套程序
时间: 2024-03-03 11:53:11 浏览: 30
好的,以下是两个简单的 Qt 程序,分别是发送端和接收端,可以实现将 /home/nvidia/a.jpg 文件通过 TCP 发送到目标主机并在接收端接收并保存。
发送端代码:
```cpp
#include <QtNetwork>
#include <QFile>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 创建 TCP Socket
QTcpSocket socket;
// 连接目标主机
socket.connectToHost("192.168.1.201", 22222);
// 等待连接建立
if (!socket.waitForConnected()) {
qCritical() << "Failed to connect to server.";
return 1;
}
// 打开文件
QFile file("/home/nvidia/a.jpg");
if (!file.open(QIODevice::ReadOnly)) {
qCritical() << "Failed to open file.";
return 1;
}
// 读取文件内容
QByteArray data = file.readAll();
// 发送数据
qint64 bytesSent = socket.write(data);
if (bytesSent < data.size()) {
qCritical() << "Failed to send all data.";
return 1;
}
// 关闭 Socket
socket.close();
return 0;
}
```
接收端代码:
```cpp
#include <QtNetwork>
#include <QFile>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 创建 TCP Server
QTcpServer server;
if (!server.listen(QHostAddress::Any, 22222)) {
qCritical() << "Failed to start server.";
return 1;
}
// 等待连接
if (!server.waitForNewConnection()) {
qCritical() << "Failed to accept new connection.";
return 1;
}
// 获取连接
QTcpSocket *clientSocket = server.nextPendingConnection();
if (!clientSocket) {
qCritical() << "Failed to get client socket.";
return 1;
}
// 创建文件
QFile file("received.jpg");
if (!file.open(QIODevice::WriteOnly)) {
qCritical() << "Failed to create file.";
return 1;
}
// 接收数据
while (clientSocket->bytesAvailable() > 0) {
QByteArray data = clientSocket->readAll();
file.write(data);
}
// 关闭 Socket 和文件
clientSocket->close();
file.close();
return 0;
}
```
将以上代码保存分别为 sender.cpp 和 receiver.cpp,并在命令行中执行以下命令编译:
```
qmake -project
qmake
make
```
然后分别运行生成的两个可执行文件即可。
相关推荐
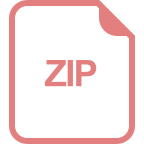
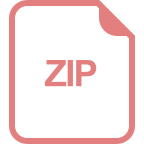















