#include<iostream> using namespace std; class Box { private: double length; public: Box(double l = 0.0):length(l){} void show() { double area = 0.0; double volunth = 0.0; cout << "该立方体的表面积为:" << area = 6 * length * length ; cout << "该立方体的体积为:" << volunth = length * length * length; } }; int main() { Box b(1); b.show; return 0; }这么改
时间: 2024-01-07 21:05:00 浏览: 35
您需要将 `b.show;` 改为 `b.show();`,以调用 `show()` 函数。修改后的代码如下:
```
#include<iostream>
using namespace std;
class Box {
private:
double length;
public:
Box(double l = 0.0):length(l){}
void show() {
double area = 0.0;
double volume = 0.0;
area = 6 * length * length ;
volume = length * length * length;
cout << "该立方体的表面积为:" << area << endl;
cout << "该立方体的体积为:" << volume << endl;
}
};
int main() {
Box b(1);
b.show();
return 0;
}
```
此时运行程序会输出该立方体的表面积和体积。
相关问题
#include<iostream> #include<cstdio> using namespace std;是什么意思
#include<iostream> #include<cstdio> using namespace std; 是C++中的预处理指令,用于引入头文件和命名空间。
1. #include<iostream> 是引入iostream头文件,其中包含了输入输出流的定义,例如cout和cin等。
2. #include<cstdio> 是引入cstdio头文件,其中包含了C语言标准输入输出函数的定义,例如printf和scanf等。
3. using namespace std; 是使用std命名空间,std是C++标准库的命名空间,其中包含了很多常用的函数和类。
这些预处理指令的作用是为了在程序中能够使用输入输出流和标准库函数,使得程序更加方便和简洁。
#include<iostream>using namespace std;
这是一个C++的代码片段,它包含了iostream头文件和使用了命名空间std。iostream头文件提供了输入输出流的功能,而using namespace std;语句则是为了方便使用std命名空间中的函数和对象,避免每次都需要写std::。
以下是一个简单的示例代码,展示了如何使用iostream头文件和命名空间std来输出一句话:
```cpp
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
```
这段代码会输出"Hello, World!",endl是一个特殊的控制符,用于在输出流中插入一个换行符。
相关推荐
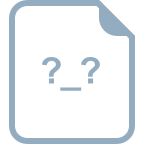












