C语言实现遗传算法的代码
时间: 2023-09-17 20:13:33 浏览: 40
以下是一个简单的C语言实现遗传算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <time.h>
#define POP_SIZE 100 // 种群大小
#define CHROM_LEN 16 // 染色体长度
#define MAX_GEN 500 // 最大迭代次数
#define P_CROSSOVER 0.8 // 交叉概率
#define P_MUTATION 0.1 // 变异概率
#define ELITIST 1 // 是否保留最优解
// 染色体结构体
typedef struct {
int gene[CHROM_LEN]; // 基因序列
double fitness; // 适应度值
} Chromosome;
// 种群结构体
typedef struct {
Chromosome chrom[POP_SIZE]; // 染色体数组
double best_fitness; // 最优解适应度值
int best_chrom[CHROM_LEN]; // 最优解基因序列
} Population;
// 初始化种群
void init_population(Population *pop) {
int i, j;
for (i = 0; i < POP_SIZE; i++) {
for (j = 0; j < CHROM_LEN; j++) {
pop->chrom[i].gene[j] = rand() % 2;
}
}
}
// 计算染色体适应度值
double calc_fitness(Chromosome chrom) {
int i;
double x = 0;
for (i = 0; i < CHROM_LEN; i++) {
x += chrom.gene[i] * pow(2, CHROM_LEN - i - 1);
}
return x * x;
}
// 计算种群适应度值和最优解
void calc_population_fitness(Population *pop) {
int i;
pop->best_fitness = 0;
for (i = 0; i < POP_SIZE; i++) {
pop->chrom[i].fitness = calc_fitness(pop->chrom[i]);
if (pop->chrom[i].fitness > pop->best_fitness) {
pop->best_fitness = pop->chrom[i].fitness;
memcpy(pop->best_chrom, pop->chrom[i].gene, sizeof(int) * CHROM_LEN);
}
}
}
// 选择操作:轮盘赌算法
void selection(Population *pop, Chromosome *parent1, Chromosome *parent2) {
int i;
double sum_fitness = 0;
double r1 = (double) rand() / RAND_MAX;
double r2 = (double) rand() / RAND_MAX;
for (i = 0; i < POP_SIZE; i++) {
sum_fitness += pop->chrom[i].fitness;
if (sum_fitness >= r1 * pop->best_fitness) {
memcpy(parent1, &pop->chrom[i], sizeof(Chromosome));
break;
}
}
sum_fitness = 0;
for (i = 0; i < POP_SIZE; i++) {
sum_fitness += pop->chrom[i].fitness;
if (sum_fitness >= r2 * pop->best_fitness) {
memcpy(parent2, &pop->chrom[i], sizeof(Chromosome));
break;
}
}
}
// 交叉操作:单点交叉
void crossover(Chromosome *parent1, Chromosome *parent2, Chromosome *child1, Chromosome *child2) {
int i;
double r = (double) rand() / RAND_MAX;
if (r <= P_CROSSOVER) {
int point = rand() % CHROM_LEN;
for (i = 0; i < point; i++) {
child1->gene[i] = parent1->gene[i];
child2->gene[i] = parent2->gene[i];
}
for (i = point; i < CHROM_LEN; i++) {
child1->gene[i] = parent2->gene[i];
child2->gene[i] = parent1->gene[i];
}
} else {
memcpy(child1, parent1, sizeof(Chromosome));
memcpy(child2, parent2, sizeof(Chromosome));
}
}
// 变异操作:单点变异
void mutation(Chromosome *chrom) {
int i;
double r = (double) rand() / RAND_MAX;
if (r <= P_MUTATION) {
int point = rand() % CHROM_LEN;
chrom->gene[point] = 1 - chrom->gene[point];
}
}
// 遗传算法主函数
void genetic_algorithm() {
int i, j;
Population pop;
Chromosome parent1, parent2, child1, child2;
srand(time(NULL));
init_population(&pop);
calc_population_fitness(&pop);
for (i = 0; i < MAX_GEN; i++) {
Population new_pop;
new_pop.best_fitness = 0;
for (j = 0; j < POP_SIZE; j += 2) {
selection(&pop, &parent1, &parent2);
crossover(&parent1, &parent2, &child1, &child2);
mutation(&child1);
mutation(&child2);
child1.fitness = calc_fitness(child1);
child2.fitness = calc_fitness(child2);
if (ELITIST) {
if (child1.fitness > pop.best_fitness) {
pop.best_fitness = child1.fitness;
memcpy(pop.best_chrom, child1.gene, sizeof(int) * CHROM_LEN);
}
if (child2.fitness > pop.best_fitness) {
pop.best_fitness = child2.fitness;
memcpy(pop.best_chrom, child2.gene, sizeof(int) * CHROM_LEN);
}
if (child1.fitness > new_pop.best_fitness) {
new_pop.best_fitness = child1.fitness;
}
if (child2.fitness > new_pop.best_fitness) {
new_pop.best_fitness = child2.fitness;
}
}
memcpy(&new_pop.chrom[j], &child1, sizeof(Chromosome));
memcpy(&new_pop.chrom[j + 1], &child2, sizeof(Chromosome));
}
if (!ELITIST) {
calc_population_fitness(&new_pop);
if (new_pop.best_fitness > pop.best_fitness) {
pop.best_fitness = new_pop.best_fitness;
memcpy(pop.best_chrom, new_pop.chrom[new_pop.best_fitness].gene, sizeof(int) * CHROM_LEN);
}
}
memcpy(&pop, &new_pop, sizeof(Population));
}
printf("Best fitness: %lf\n", pop.best_fitness);
printf("Best chromosome: ");
for (i = 0; i < CHROM_LEN; i++) {
printf("%d", pop.best_chrom[i]);
}
printf("\n");
}
int main() {
genetic_algorithm();
return 0;
}
```
以上是一个简单的遗传算法的C语言实现,可以用于解决某些优化问题。需注意的是,遗传算法的效果受到参数的影响,需要根据问题的具体情况进行调整。
相关推荐
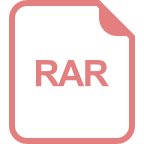
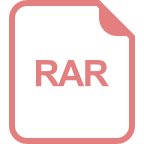
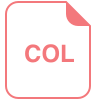
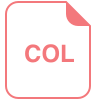
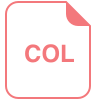
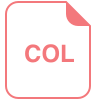










