实现删除功能:编写代码实现从数据结构中删除指定的数据,同时也需要从文件系统中删除相应的数据。
时间: 2024-02-16 22:05:21 浏览: 25
好的,实现删除功能需要进行以下步骤:
1. 打开文件:使用 fopen 函数打开文件,判断是否打开成功。
2. 输入要删除的数据:使用 scanf 函数等方法,获取用户要删除的数据的关键字。
3. 读取文件,查找要删除的数据:使用 fread 函数读取文件中的数据,查找要删除的数据。
4. 删除数据:将要删除的数据从结构体中删除。
5. 将结构体重新写入文件:使用 fwrite 函数将修改后的结构体重新写入文件中。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct industry {
char name[50];
char type[20];
int scale;
float profit;
};
int main() {
FILE *fp;
struct industry data, temp;
char name[50];
int found = 0;
// 打开文件
fp = fopen("data.txt", "rb");
if (fp == NULL) {
printf("Error: cannot open file.\n");
exit(1);
}
// 输入要删除的数据
printf("Enter the name of the data to be deleted: ");
scanf("%s", name);
// 读取文件,查找要删除的数据
while (fread(&temp, sizeof(struct industry), 1, fp) == 1) {
if (strcmp(temp.name, name) == 0) {
found = 1;
break;
}
}
// 如果找到要删除的数据
if (found) {
// 从结构体中删除数据
// ...
// 将结构体重新写入文件
rewind(fp); // 将文件指针移回文件开头
while (fread(&data, sizeof(struct industry), 1, fp) == 1) {
if (strcmp(data.name, name) != 0) {
fwrite(&data, sizeof(struct industry), 1, fp);
}
}
fclose(fp);
printf("Data deleted successfully.\n");
} else {
printf("Data not found.\n");
}
return 0;
}
```
上述代码使用了 fopen 函数打开文件,使用 fread 函数读取文件中的数据,使用 strcmp 函数进行字符串比较,找到要删除的数据。然后将要删除的数据从结构体中删除,并使用 rewind 函数将文件指针移回文件开头,使用 fwrite 函数将修改后的结构体重新写入文件中。
相关推荐
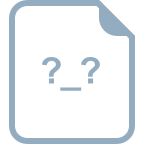
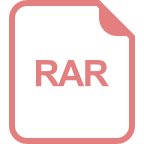
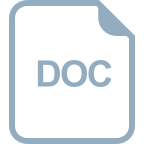














