、整合Spring+Mybatis框架并实现增删改查功能 2、在实验一的基础上,整合MyBatis,要求数据从数据库查询 3、导入jdbc.properties属性文件 4、导入log4j.xml属性文件 5、编写application-context.xml核心配置文件 6、创建实体类Student(id, name,studentNo, age) 其他属性可自行补充 7、创建对应的Mapper文件并编写SQL,实现增删改查功能 8、创建单元测试类并实现CRUD各项功能
时间: 2024-02-07 13:02:05 浏览: 30
好的,你的问题是如何整合Spring+Mybatis框架并实现增删改查功能,我来为你解答。
首先,我们需要在项目中引入Spring和MyBatis的依赖,可以使用Maven或者Gradle管理依赖。这里以Maven为例,引入如下依赖:
```xml
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.5</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.5</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.5</version>
</dependency>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.6</version>
</dependency>
<!-- Database Driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
```
接下来,我们需要在Spring配置文件中配置MyBatis,这里以application-context.xml为例:
```xml
<!-- 数据源配置 -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${jdbc.driverClassName}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
<!-- MyBatis配置 -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.example.demo.model"/>
<property name="mapperLocations" value="classpath:mapper/*.xml"/>
</bean>
<!-- Mapper扫描 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.example.demo.mapper"/>
</bean>
```
在这个配置文件中,我们配置了数据源和MyBatis的SqlSessionFactory,同时指定了Mapper的xml文件所在的位置和Mapper扫描的包。
接下来,我们创建实体类Student:
```java
public class Student {
private int id;
private String name;
private String studentNo;
private int age;
// getter和setter方法省略
}
```
然后,我们创建对应的Mapper文件,并编写SQL,实现增删改查功能,这里以StudentMapper.xml为例:
```xml
<mapper namespace="com.example.demo.mapper.StudentMapper">
<resultMap id="studentMap" type="com.example.demo.model.Student">
<id property="id" column="id"/>
<result property="name" column="name"/>
<result property="studentNo" column="student_no"/>
<result property="age" column="age"/>
</resultMap>
<select id="getStudentById" resultMap="studentMap">
SELECT id,name,student_no,age FROM student WHERE id=#{id}
</select>
<select id="getAllStudents" resultMap="studentMap">
SELECT id,name,student_no,age FROM student
</select>
<insert id="insertStudent" useGeneratedKeys="true" keyProperty="id">
INSERT INTO student(name,student_no,age) VALUES(#{name},#{studentNo},#{age})
</insert>
<update id="updateStudent">
UPDATE student SET name=#{name},student_no=#{studentNo},age=#{age} WHERE id=#{id}
</update>
<delete id="deleteStudentById">
DELETE FROM student WHERE id=#{id}
</delete>
</mapper>
```
在Mapper中,我们定义了查询单个学生和查询所有学生的方法,以及插入、更新和删除学生的方法。
最后,我们创建单元测试类并实现CRUD各项功能:
```java
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = {"classpath:application-context.xml"})
public class StudentMapperTest {
@Autowired
private StudentMapper studentMapper;
@Test
public void testGetStudentById() {
Student student = studentMapper.getStudentById(1);
assertNotNull(student);
assertEquals("Tom", student.getName());
}
@Test
public void testGetAllStudents() {
List<Student> students = studentMapper.getAllStudents();
assertNotNull(students);
assertEquals(2, students.size());
}
@Test
public void testInsertStudent() {
Student student = new Student();
student.setName("Lucy");
student.setStudentNo("001");
student.setAge(18);
int result = studentMapper.insertStudent(student);
assertEquals(1, result);
}
@Test
public void testUpdateStudent() {
Student student = new Student();
student.setId(1);
student.setName("Tommy");
student.setStudentNo("002");
student.setAge(20);
int result = studentMapper.updateStudent(student);
assertEquals(1, result);
}
@Test
public void testDeleteStudentById() {
int result = studentMapper.deleteStudentById(2);
assertEquals(1, result);
}
}
```
在单元测试类中,我们注入了StudentMapper,并分别测试了查询单个学生、查询所有学生、插入学生、更新学生和删除学生的功能。
这就是整合Spring+Mybatis框架并实现增删改查功能的步骤,希望能对你有所帮助。
相关推荐
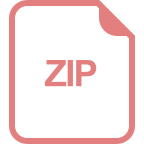
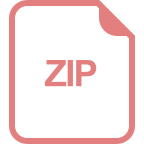














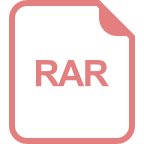