c++实现dsa
时间: 2023-06-29 12:04:25 浏览: 122
C++ 是一种非常适合实现数据结构和算法的编程语言。以下是一个简单的示例,展示了如何使用 C++ 实现数据结构和算法。
1. 实现一个链表
```cpp
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
int main() {
ListNode *head = new ListNode(1);
ListNode *p = head;
p->next = new ListNode(2);
p = p->next;
p->next = new ListNode(3);
p = p->next;
p->next = new ListNode(4);
p = p->next;
p->next = new ListNode(5);
p = head;
while (p != NULL) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
return 0;
}
```
2. 实现一个栈
```cpp
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> st;
st.push(1);
st.push(2);
st.push(3);
st.push(4);
st.push(5);
while (!st.empty()) {
cout << st.top() << " ";
st.pop();
}
cout << endl;
return 0;
}
```
3. 实现一个队列
```cpp
#include <iostream>
#include <queue>
using namespace std;
int main() {
queue<int> q;
q.push(1);
q.push(2);
q.push(3);
q.push(4);
q.push(5);
while (!q.empty()) {
cout << q.front() << " ";
q.pop();
}
cout << endl;
return 0;
}
```
4. 实现一个排序算法
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> nums = {5, 2, 3, 4, 1};
sort(nums.begin(), nums.end());
for (auto num : nums) {
cout << num << " ";
}
cout << endl;
return 0;
}
```
这只是一些简单的示例,C++ 中有许多更复杂的数据结构和算法可以实现。
阅读全文
相关推荐
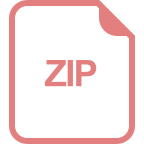
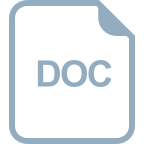
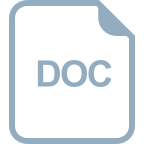






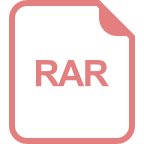
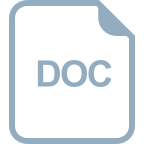
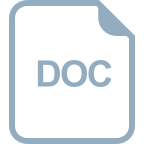
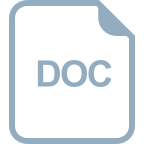
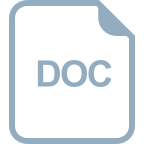

