利用链表编写下列程序 对学生的成绩信息进行管理, 其中包括学生信息, 课程信息,和选课信息。学生信息包括:学号、姓名、性别和地址。 课程信息包括课程号,课程名,学分,学时。 选课信息包括学号, 姓名, 课程号,课程名, 学期, 和成绩。
时间: 2023-12-03 18:05:08 浏览: 136
以下是一个简单的链表程序,实现了上述功能:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义学生信息结构体
struct Student {
string id; // 学号
string name; // 姓名
char gender; // 性别
string address; // 地址
};
// 定义课程信息结构体
struct Course {
string id; // 课程号
string name; // 课程名
int credit; // 学分
int hours; // 学时
};
// 定义选课信息结构体
struct Select {
string sid; // 学号
string sname; // 姓名
string cid; // 课程号
string cname; // 课程名
string term; // 学期
int score; // 成绩
};
// 定义链表结构体
struct Node {
Student stu;
Course cour;
Select sel;
Node* next;
};
// 添加学生信息到链表
void addStudent(Node* head) {
Node* cur = head;
while (cur->next != nullptr) {
cur = cur->next;
}
Student stu;
cout << "请输入学生信息:" << endl;
cout << "学号:";
cin >> stu.id;
cout << "姓名:";
cin >> stu.name;
cout << "性别:";
cin >> stu.gender;
cout << "地址:";
cin >> stu.address;
Node* node = new Node{stu, Course(), Select(), nullptr};
cur->next = node;
cout << "添加成功!" << endl;
}
// 添加课程信息到链表
void addCourse(Node* head) {
Node* cur = head;
while (cur->next != nullptr) {
cur = cur->next;
}
Course cour;
cout << "请输入课程信息:" << endl;
cout << "课程号:";
cin >> cour.id;
cout << "课程名:";
cin >> cour.name;
cout << "学分:";
cin >> cour.credit;
cout << "学时:";
cin >> cour.hours;
Node* node = new Node{Student(), cour, Select(), nullptr};
cur->next = node;
cout << "添加成功!" << endl;
}
// 添加选课信息到链表
void addSelect(Node* head) {
Node* cur = head;
while (cur->next != nullptr) {
cur = cur->next;
}
Select sel;
cout << "请输入选课信息:" << endl;
cout << "学号:";
cin >> sel.sid;
cout << "姓名:";
cin >> sel.sname;
cout << "课程号:";
cin >> sel.cid;
cout << "课程名:";
cin >> sel.cname;
cout << "学期:";
cin >> sel.term;
cout << "成绩:";
cin >> sel.score;
Node* node = new Node{Student(), Course(), sel, nullptr};
cur->next = node;
cout << "添加成功!" << endl;
}
// 显示所有学生信息
void showStudents(Node* head) {
Node* cur = head->next;
while (cur != nullptr) {
cout << "学号:" << cur->stu.id << endl;
cout << "姓名:" << cur->stu.name << endl;
cout << "性别:" << cur->stu.gender << endl;
cout << "地址:" << cur->stu.address << endl;
cout << endl;
cur = cur->next;
}
}
// 显示所有课程信息
void showCourses(Node* head) {
Node* cur = head->next;
while (cur != nullptr) {
cout << "课程号:" << cur->cour.id << endl;
cout << "课程名:" << cur->cour.name << endl;
cout << "学分:" << cur->cour.credit << endl;
cout << "学时:" << cur->cour.hours << endl;
cout << endl;
cur = cur->next;
}
}
// 显示所有选课信息
void showSelects(Node* head) {
Node* cur = head->next;
while (cur != nullptr) {
cout << "学号:" << cur->sel.sid << endl;
cout << "姓名:" << cur->sel.sname << endl;
cout << "课程号:" << cur->sel.cid << endl;
cout << "课程名:" << cur->sel.cname << endl;
cout << "学期:" << cur->sel.term << endl;
cout << "成绩:" << cur->sel.score << endl;
cout << endl;
cur = cur->next;
}
}
int main() {
// 创建链表头结点
Node* head = new Node{Student(), Course(), Select(), nullptr};
// 显示菜单
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加学生信息" << endl;
cout << "2. 添加课程信息" << endl;
cout << "3. 添加选课信息" << endl;
cout << "4. 显示所有学生信息" << endl;
cout << "5. 显示所有课程信息" << endl;
cout << "6. 显示所有选课信息" << endl;
cout << "0. 退出程序" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
addStudent(head);
break;
case 2:
addCourse(head);
break;
case 3:
addSelect(head);
break;
case 4:
showStudents(head);
break;
case 5:
showCourses(head);
break;
case 6:
showSelects(head);
break;
case 0:
return 0;
default:
cout << "无效的选择!" << endl;
break;
}
}
}
```
阅读全文
相关推荐

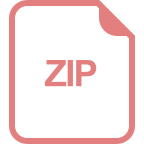
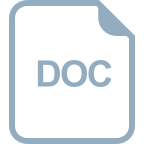
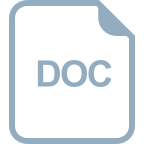











