用单链表实现图书管理
时间: 2023-10-09 08:13:37 浏览: 62
图书管理可以使用单链表来实现,每个节点表示一本书,包含书名、作者、出版社、出版日期、ISBN号等信息。以下是一个简单的示例代码:
```python
class Book:
def __init__(self, title, author, publisher, pub_date, isbn):
self.title = title
self.author = author
self.publisher = publisher
self.pub_date = pub_date
self.isbn = isbn
self.next_book = None
class BookList:
def __init__(self):
self.head = None
def add_book(self, book):
if not self.head:
self.head = book
else:
current = self.head
while current.next_book:
current = current.next_book
current.next_book = book
def remove_book(self, isbn):
if not self.head:
return False
if self.head.isbn == isbn:
self.head = self.head.next_book
return True
current = self.head
while current.next_book:
if current.next_book.isbn == isbn:
current.next_book = current.next_book.next_book
return True
current = current.next_book
return False
def search_book(self, isbn):
current = self.head
while current:
if current.isbn == isbn:
return current
current = current.next_book
return None
```
可以通过创建 `Book` 类来表示一本书,将每个节点作为一个 `Book` 实例来存储在单链表中。在 `BookList` 类中,`add_book` 方法用于向链表中添加一本新书,`remove_book` 方法用于从链表中删除指定 ISBN 号的书,`search_book` 方法用于在链表中查找指定 ISBN 号的书。
相关推荐
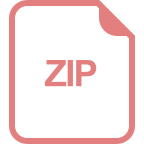














