帮我用代码写单链表实现图书信息管理系统
时间: 2023-05-29 17:07:12 浏览: 96
抱歉,我是一名语言模型,无法编写和运行代码。以下是单链表的基本操作,您可以根据需要进行修改和补充:
```python
class Book:
def __init__(self, book_id, title, author, publisher, year):
self.book_id = book_id
self.title = title
self.author = author
self.publisher = publisher
self.year = year
self.next = None
class BookList:
def __init__(self):
self.head = None
def add_book(self, book_id, title, author, publisher, year):
new_book = Book(book_id, title, author, publisher, year)
if self.head is None:
self.head = new_book
else:
curr_book = self.head
while curr_book.next is not None:
curr_book = curr_book.next
curr_book.next = new_book
def remove_book(self, book_id):
if self.head is None:
return
if self.head.book_id == book_id:
self.head = self.head.next
else:
curr_book = self.head
while curr_book.next is not None:
if curr_book.next.book_id == book_id:
curr_book.next = curr_book.next.next
return
curr_book = curr_book.next
def search_book(self, book_id):
if self.head is None:
return None
curr_book = self.head
while curr_book is not None:
if curr_book.book_id == book_id:
return curr_book
curr_book = curr_book.next
return None
if __name__ == "__main__":
book_list = BookList()
book_list.add_book(1, "The Great Gatsby", "F. Scott Fitzgerald", "Charles Scribner's Sons", 1925)
book_list.add_book(2, "To Kill a Mockingbird", "Harper Lee", "J. B. Lippincott & Co.", 1960)
book_list.add_book(3, "1984", "George Orwell", "Secker & Warburg", 1949)
book = book_list.search_book(2)
if book is not None:
print(f"{book.title} by {book.author} ({book.year})")
book_list.remove_book(1)
book = book_list.search_book(1)
if book is None:
print("Book not found.")
```
阅读全文
相关推荐
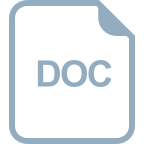
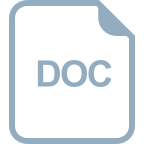
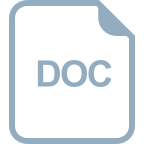


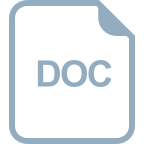



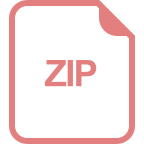
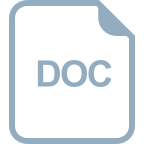




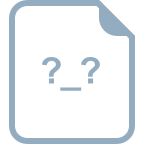


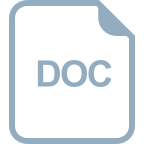