定义一个学生类,数据成员包括学号,姓名,年龄 ,三科成绩,包括若干成员函数。编写主程序完成对学生数据的赋值。要求,用构造函数完成输入 定义对象数组完成相应功能,编写一个函数,以学号为参数,在数组中查找学号为n的学生,并返回其全部信息。
时间: 2023-06-15 13:03:18 浏览: 119
以下是一个可能的学生类的定义和主程序的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(int id_, string name_, int age_, int score1_, int score2_, int score3_) :
id(id_), name(name_), age(age_), score1(score1_), score2(score2_), score3(score3_) {}
// 构造函数,用于输入学生信息
void print() {
cout << "ID: " << id << endl;
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Score1: " << score1 << endl;
cout << "Score2: " << score2 << endl;
cout << "Score3: " << score3 << endl;
}
// 打印学生信息
int get_id() {
return id;
}
// 获取学生学号
private:
int id;
string name;
int age;
int score1, score2, score3;
};
Student find_student(Student students[], int n, int id) {
for (int i = 0; i < n; i++) {
if (students[i].get_id() == id) {
return students[i];
}
}
// 如果没有找到对应学生,返回一个默认构造的学生对象
return Student(-1, "", -1, -1, -1, -1);
}
int main() {
const int N = 3;
Student students[N] = {
Student(1001, "Alice", 18, 90, 80, 85),
Student(1002, "Bob", 19, 85, 92, 88),
Student(1003, "Charlie", 18, 95, 85, 90)
};
int id;
cout << "Enter the student ID to search: ";
cin >> id;
Student result = find_student(students, N, id);
if (result.get_id() == -1) {
cout << "Student not found!" << endl;
}
else {
result.print();
}
return 0;
}
```
这个程序中,我们首先定义了一个 `Student` 类,包含了学生的基本信息和三科成绩。构造函数用于输入学生信息,`print` 函数用于打印学生信息,`get_id` 函数用于获取学生学号。然后我们定义了一个 `find_student` 函数,用于在学生数组中查找特定学号的学生,并返回其信息。最后在主程序中定义了一个长度为 3 的学生对象数组,并且调用 `find_student` 函数查找特定学号的学生并打印其信息。
阅读全文
相关推荐
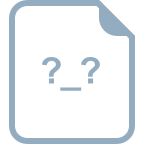
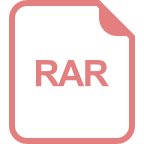
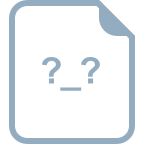

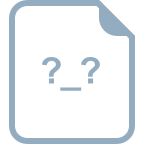













