自己设计的KString类怎么重载<<运算符,让cout可以将其输出
时间: 2024-05-15 08:15:18 浏览: 63
要重载<<运算符,让cout可以输出KString类的对象,需要在KString类中添加一个友元函数。
下面是KString类的代码示例:
```cpp
#include <iostream>
#include <cstring>
class KString {
public:
KString(const char* str = "") {
size = strlen(str);
data = new char[size + 1];
strcpy(data, str);
}
// 拷贝构造函数
KString(const KString& other) {
size = other.size;
data = new char[size + 1];
strcpy(data, other.data);
}
// 析构函数
~KString() {
delete[] data;
}
// 重载赋值运算符
KString& operator=(const KString& other) {
if (this != &other) {
delete[] data;
size = other.size;
data = new char[size + 1];
strcpy(data, other.data);
}
return *this;
}
// 重载+运算符
KString operator+(const KString& other) {
char* temp = new char[size + other.size + 1];
strcpy(temp, data);
strcat(temp, other.data);
KString result(temp);
delete[] temp;
return result;
}
// 重载<<运算符
friend std::ostream& operator<<(std::ostream& os, const KString& str) {
os << str.data;
return os;
}
private:
char* data;
int size;
};
```
在KString类中,我们添加了一个友元函数`operator<<`,它接受一个ostream对象和一个KString对象作为参数。在函数中,我们将KString对象的data成员输出到ostream对象中,然后返回ostream对象,这样就可以方便地使用cout输出KString对象了。
下面是使用KString类的示例代码:
```cpp
int main() {
KString str1("Hello"), str2("World");
KString str3 = str1 + str2;
std::cout << str1 << " " << str2 << "\n";
std::cout << "Concatenation: " << str3 << "\n";
return 0;
}
```
输出结果为:
```
Hello World
Concatenation: HelloWorld
```
阅读全文
相关推荐




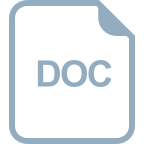
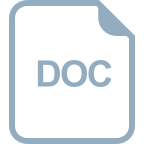
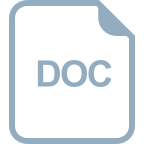







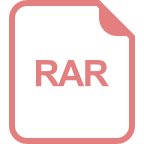