1)重载运算符“+”,“-” (2)重载运算符cout“<<”中的“<<” (3)重载运算符“>”,“<”和“==”,依据复数的模进行判断。
时间: 2023-11-05 15:30:10 浏览: 30
1)重载运算符“ ”,“-”:可以重载这两个运算符,对应的功能分别是加法和减法。重载后,可以方便地对两个复数进行加减运算。
2)重载运算符cout“<<”中的“<<”:可以在类中定义一个友元函数,函数名为"operator<<",该函数的参数是流类型和一个复数对象。这样,就可以在输出流中使用"<<""运算符输出复数对象的实部和虚部。
3)重载运算符“>”,“<”和“==”,依据复数的模进行判断:可以定义一个重载"=="运算符的函数,比较两个复数的模是否相等。再定义一个重载">"和"<"运算符的函数,根据两个复数的模大小,返回布尔值。三个运算符实现后,对于复数对象的比较就可以方便容易地进行了。
相关问题
cout << f1+f2 << endl;需要如何重载运算符
如果 f1 和 f2 是自定义的类型,比如 Fraction(分数) 类型,那么可以重载加号运算符来实现 f1+f2 的操作。具体实现如下:
```C++
class Fraction {
public:
int numerator; // 分子
int denominator; // 分母
Fraction operator+(const Fraction& other) const { // 重载加号运算符
int lcm = denominator * other.denominator / gcd(denominator, other.denominator); // 求最小公倍数
int numerator_sum = numerator * lcm / denominator + other.numerator * lcm / other.denominator; // 加法运算
return {numerator_sum, lcm}; // 返回结果
}
};
// 在主函数中使用:
Fraction f1{1, 2};
Fraction f2{1, 3};
cout << (f1 + f2).numerator << "/" << (f1 + f2).denominator << endl;
```
上述代码中,我们重载了 Fraction 类的加号运算符,返回一个新的 Fraction 对象,表示两个分数相加的结果。在主函数中,我们先创建了两个分数 f1 和 f2,然后使用重载的加号运算符计算它们的和,并输出结果。
需要注意的是,重载运算符时需要遵循一些规则,比如:
- 运算符函数必须是类的成员函数或者是友元函数;
- 运算符函数的参数个数与操作数个数相同;
- 运算符函数的返回值类型与操作数类型相同,或者是可以隐式转换成操作数类型的类型;
- 等等。
如果你想深入了解运算符重载的规则和实现方法,可以参考相关的 C++ 教程或者书籍。
cout << f1+f2 << endl;需要如何重载+运算符
如果 `f1` 和 `f2` 是自定义的类,我们可以通过重载 `+` 运算符来实现 `cout << f1+f2 << endl;` 的功能。
要重载 `+` 运算符,可以在类中定义一个成员函数或者定义一个全局函数。以下是定义一个全局函数来重载 `+` 运算符的示例:
```c++
class Fraction {
public:
int numerator, denominator;
Fraction(int n, int d) : numerator(n), denominator(d) {}
};
Fraction operator+(const Fraction& f1, const Fraction& f2) {
int n = f1.numerator * f2.denominator + f2.numerator * f1.denominator;
int d = f1.denominator * f2.denominator;
return Fraction(n, d);
}
```
在上面的示例中,我们定义了一个名为 `operator+` 的全局函数来重载 `+` 运算符。该函数接受两个参数,分别为 `Fraction` 类型的对象 `f1` 和 `f2`,并返回一个新的 `Fraction` 对象,其值等于 `f1` 和 `f2` 相加的结果。
使用重载后的 `+` 运算符时,可以直接像使用内置类型一样使用:
```c++
Fraction f1(1, 2), f2(2, 3);
cout << f1 + f2 << endl;
```
输出结果为:
```
7/6
```
相关推荐
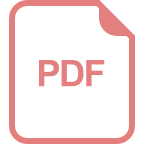
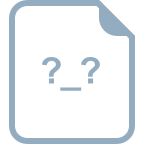
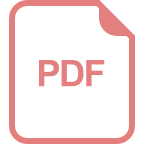













