#include<stdio.h> int Count_Digit( const int N) { int a=0,b,c=N; if(c<0)c=-c; while(c!=0) { a=c%10; if(a%2!=0)b+=a; c=c/10; } return b; } int main( ) { int N; scanf("%d",&N); printf("%d",Count_Digit(N)); return 0; }
时间: 2024-01-15 14:03:46 浏览: 38
这是一个 C 语言的实现,与我之前给出的 Python 实现类似,也是遍历每一位数字并判断是否为奇数,最后累加奇数的和并返回。下面是这个函数的具体实现:
```c
#include<stdio.h>
int Count_Digit(const int N) {
int a = 0, b = 0, c = N;
if (c < 0) c = -c; // 处理负数的情况
while (c != 0) {
a = c % 10;
if (a % 2 != 0) b += a;
c = c / 10;
}
return b;
}
int main() {
int N;
scanf("%d", &N);
printf("%d", Count_Digit(N));
return 0;
}
```
需要注意的是,这个函数里面有一个变量 b,用来累加奇数的和。在函数开始时,需要将 b 初始化为 0。此外,还要处理输入的整数 N 可能是负数的情况。如果 N 是负数,需要先将其取绝对值再进行遍历和计算。
这个函数的时间复杂度为 O(log N),因为它需要遍历整数的每一位数字。
相关问题
#include <stdio.h> #include <stdlib.h> #include <ctype.h> #define MAX_FILENAME_LEN 256 #define MAX_LINE_LEN 1024 struct CountResult { int word_count; int letter_count; int digit_count; int space_count; int newline_count; int punct_count; int other_count; }; struct CountResult count_chars(const char* filename) { struct CountResult result = { 0, 0, 0, 0, 0, 0, 0 }; FILE* fp; char line[MAX_LINE_LEN]; int c; int i;
这段代码定义了一个名为 CountResult 的结构体,包含了单词数量、字母数量、数字数量、空格数量、换行数量、标点符号数量和其他字符数量这七个成员变量。然后定义了一个名为 count_chars 的函数,该函数接受一个文件名作为参数,返回一个 CountResult 结构体类型的结果。
在函数内部,首先定义了一个名为 result 的 CountResult 结构体变量,并将其所有成员变量初始化为 0。然后打开了该文件名对应的文件,并逐行读取文件内容。在每一行中,函数统计了字母、数字、空格、换行、标点符号和其他字符的数量,并将结果累加到 result 结构体变量的相应成员变量中。
最后,函数返回了统计结果 result。
可以告诉我接下来这个程序的命令行参数命名规则吗#include <stdio.h> #include <stdlib.h> #include <ctype.h> #define MAX_FILENAME_LEN 256 #define MAX_LINE_LEN 1024 struct CountResult { int word_count; int letter_count; int digit_count; int space_count; int newline_count; int punct_count; int other_count; }; struct CountResult count_chars(const char* filename) { struct CountResult result = { 0, 0, 0, 0, 0, 0, 0 }; FILE* fp; char line[MAX_LINE_LEN]; int c; int i; fopen(filename,"r"); if (fp == NULL) { fprintf(stderr, "Error: cannot open file '%s'\n", filename); exit(1); } while (fgets(line, MAX_LINE_LEN, fp) != NULL) { for (i = 0; line[i] != '\0'; i++) { c = line[i]; if (isalpha(c)) { result.letter_count++; } else if (isdigit(c)) { result.digit_count++; } else if (isspace(c)) { result.space_count++; if (c == '\n') { result.newline_count++; } } else if (ispunct(c)) { result.punct_count++; } else { result.other_count++; } } char* p = line; while (*p != '\0') { while (*p != '\0' && !isalnum(*p)) { p++; } if (*p != '\0') { result.word_count++; while (*p != '\0' && isalnum(*p)) { p++; } } } } fclose(fp); return result; }
这个程序需要接收一个文件名作为参数,用于统计该文件中的字符、单词、数字、空白符、换行符、标点符号和其他字符的数量。在命令行中使用该程序时,需要在程序名后面加上一个文件名参数,例如:
```
./count_chars.exe filename.txt
```
其中,`./count_chars.exe` 是程序名,`filename.txt` 是要统计的文件名。
相关推荐
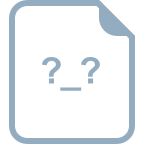
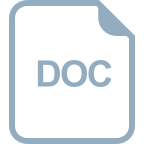
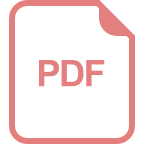












