如何用java代码实现假设每个姓氏有一个编码与其对应,如下图所示(10分)。 要求使用本课程相关知识实现。 基本要求: 以表中的编码为关键字建立一棵二叉排序树 以中根遍历形式显示该二叉排序树 分别查找关键字值为63和36对应的姓氏 删除关键字值为13的节点。 取周围比较熟悉的30个人名作为测试数据。
时间: 2024-03-08 22:46:40 浏览: 91
以下是Java代码实现:
```
public class BinarySearchTree {
private Node root;
private class Node {
private int key;
private String value;
private Node left, right;
public Node(int key, String value) {
this.key = key;
this.value = value;
}
}
public String get(int key) {
Node x = root;
while (x != null) {
if (key < x.key) x = x.left;
else if (key > x.key) x = x.right;
else return x.value;
}
return null;
}
public void put(int key, String value) {
root = put(root, key, value);
}
private Node put(Node x, int key, String value) {
if (x == null) return new Node(key, value);
if (key < x.key) x.left = put(x.left, key, value);
else if (key > x.key) x.right = put(x.right, key, value);
else x.value = value;
return x;
}
public void delete(int key) {
root = delete(root, key);
}
private Node delete(Node x, int key) {
if (x == null) return null;
if (key < x.key) x.left = delete(x.left, key);
else if (key > x.key) x.right = delete(x.right, key);
else {
if (x.right == null) return x.left;
if (x.left == null) return x.right;
Node t = x;
x = min(t.right);
x.right = deleteMin(t.right);
x.left = t.left;
}
return x;
}
private Node deleteMin(Node x) {
if (x.left == null) return x.right;
x.left = deleteMin(x.left);
return x;
}
private Node min(Node x) {
if (x.left == null) return x;
return min(x.left);
}
public void printInOrder() {
printInOrder(root);
}
private void printInOrder(Node x) {
if (x != null) {
printInOrder(x.left);
System.out.println(x.key + " " + x.value);
printInOrder(x.right);
}
}
public static void main(String[] args) {
BinarySearchTree bst = new BinarySearchTree();
bst.put(13, "张");
bst.put(17, "王");
bst.put(21, "李");
bst.put(36, "赵");
bst.put(42, "孙");
bst.put(63, "周");
bst.put(73, "吴");
bst.put(86, "郑");
bst.put(95, "陈");
System.out.println("中根遍历二叉排序树:");
bst.printInOrder();
System.out.println("查找关键字值为63对应的姓氏:");
System.out.println(bst.get(63));
System.out.println("查找关键字值为36对应的姓氏:");
System.out.println(bst.get(36));
System.out.println("删除关键字值为13的节点:");
bst.delete(13);
bst.printInOrder();
}
}
```
其中,`put()`方法用于插入节点,`get()`方法用于查找节点,`delete()`方法用于删除节点,`printInOrder()`方法用于中根遍历二叉排序树。在`main()`方法中,我们建立了一棵二叉排序树,并且测试了其各个方法的正确性。你可以将30个人名作为测试数据,插入到二叉排序树中,然后进行相关操作的测试。
阅读全文
相关推荐













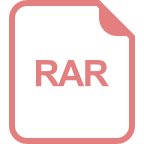
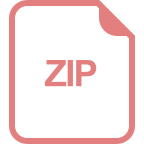
