C语言实现获取elf文件section,并将新的文件合并到section中,示例
时间: 2023-06-09 19:08:15 浏览: 104
代码可否提供?
可以的,以下是一个简单的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <elf.h>
int main(int argc, char **argv) {
if (argc < 4) {
printf("Usage: %s <elf_file> <section_name> <new_file>\n", argv[0]);
return 1;
}
const char *elf_file = argv[1];
const char *section_name = argv[2];
const char *new_file = argv[3];
FILE *f = fopen(elf_file, "rb");
if (f == NULL) {
printf("Error opening file: %s\n", elf_file);
return 1;
}
fseek(f, 0, SEEK_END);
size_t file_size = ftell(f);
fseek(f, 0, SEEK_SET);
char *elf_data = (char *)malloc(file_size);
fread(elf_data, file_size, 1, f);
fclose(f);
Elf32_Ehdr *ehdr = (Elf32_Ehdr *)elf_data;
if (memcmp(ehdr->e_ident, ELFMAG, SELFMAG) != 0) {
printf("Not an ELF file\n");
return 1;
}
Elf32_Shdr *shdr_start = (Elf32_Shdr *)(elf_data + ehdr->e_shoff);
Elf32_Shdr *shdr_strtab = shdr_start + ehdr->e_shstrndx;
char *shstrtab = elf_data + shdr_strtab->sh_offset;
int section_index = -1;
for (int i = 0; i < ehdr->e_shnum; i++) {
Elf32_Shdr *shdr = shdr_start + i;
char *section_name_at = shstrtab + shdr->sh_name;
if (strcmp(section_name_at, section_name) == 0) {
section_index = i;
break;
}
}
if (section_index == -1) {
printf("Section not found: %s\n", section_name);
return 1;
}
FILE *new_f = fopen(new_file, "rb");
if (new_f == NULL) {
printf("Error opening new file: %s\n", new_file);
return 1;
}
fseek(new_f, 0, SEEK_END);
size_t new_file_size = ftell(new_f);
fseek(new_f, 0, SEEK_SET);
char *new_data = (char *)malloc(new_file_size);
fread(new_data, new_file_size, 1, new_f);
fclose(new_f);
Elf32_Shdr *shdr = shdr_start + section_index;
char *section_data = elf_data + shdr->sh_offset;
size_t section_size = shdr->sh_size;
// Resize the section to fit the new data
if (new_file_size > section_size) {
char *new_section_data = (char *)realloc(section_data, new_file_size);
if (new_section_data == NULL) {
printf("Error reallocating memory\n");
return 1;
}
section_data = new_section_data;
memset(section_data + section_size, 0, new_file_size - section_size);
}
// Copy the contents of the new file into the section
memcpy(section_data, new_data, new_file_size);
// Update the section size
shdr->sh_size = new_file_size;
// Write the modified ELF file back to disk
FILE *out_f = fopen(elf_file, "wb");
if (out_f == NULL) {
printf("Error opening output file: %s\n", elf_file);
return 1;
}
fwrite(elf_data, file_size, 1, out_f);
fclose(out_f);
printf("Section '%s' updated in file '%s' with contents of file '%s'\n", section_name, elf_file, new_file);
return 0;
}
```
这个程序接受三个参数:ELF 文件路径,需要更新的段名和新文件路径。它打开 ELF 文件,查找指定的段,将指定的新文件的内容覆盖到该段中,然后将新的 ELF 文件写回磁盘。您可以将这个程序编译成可执行文件,然后以以下格式运行:
```
./program <elf_file> <section_name> <new_file>
```
其中 `<elf_file>` 是需要更新的 ELF 文件的路径,`<section_name>` 是需要更新的段的名字,`<new_file>` 是需要合并到段中的新文件的路径。
相关推荐
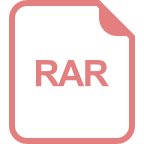
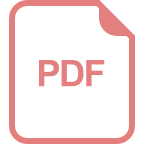
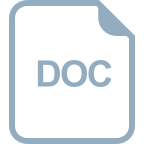














