用python实现单字符的凯撒密码加密
时间: 2023-05-21 14:02:16 浏览: 122
可以使用以下代码实现单字符的凯撒密码加密:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
if char.isupper():
result += chr((ord(char) + shift - 65) % 26 + 65)
else:
result += chr((ord(char) + shift - 97) % 26 + 97)
else:
result += char
return result
```
其中,text是要加密的文本,shift是加密的偏移量。该函数会返回加密后的文本。
例如,使用该函数加密文本"hello world",偏移量为3,可以得到加密后的文本"khoor zruog"。
注意:该加密方式并不安全,只是一种简单的加密方式。
相关问题
用python编写一个凯撒密码加密算法
好的,以下是用 Python 编写的凯撒密码加密算法示例:
```python
def caesar_encrypt(plain_text, shift):
"""凯撒密码加密函数"""
cipher_text = ""
for char in plain_text:
if char.isalpha():
# 对字母进行加密
ascii_code = ord(char) + shift
if char.isupper():
if ascii_code > ord('Z'):
ascii_code -= 26
elif ascii_code < ord('A'):
ascii_code += 26
else:
if ascii_code > ord('z'):
ascii_code -= 26
elif ascii_code < ord('a'):
ascii_code += 26
cipher_text += chr(ascii_code)
else:
# 非字母字符直接添加到密文中
cipher_text += char
return cipher_text
```
这个函数接收两个参数,第一个参数 plain_text 是要加密的明文,第二个参数 shift 是加密时的位移量。函数会遍历明文中的每个字符,对字母进行加密,非字母字符则直接添加到密文中。字母加密时会根据大小写分别处理,并根据位移量进行加密。
下面是一个示例,使用该函数进行加密:
```python
plain_text = "hello, world!"
shift = 3
cipher_text = caesar_encrypt(plain_text, shift)
print(cipher_text)
```
输出结果为:
```
khoor, zruog!
```
Python用凯撒密码加密Python字符
Python可以使用凯撒密码对字符串进行加密。凯撒密码是通过将字母移动一定的位数来实现加密和解密。在Python中,可以使用字符串模块中的ascii_lowercase和ascii_uppercase来获取小写和大写的英文字母,然后通过建立循环字母表来实现加密和解密。下面是一个示例代码:
```
import string
def caesar_cipher_encrypt(s, k):
lower = string.ascii_lowercase
upper = string.ascii_uppercase
before = string.ascii_letters
after = lower[k:] + lower[:k] + upper[k:] + upper[:k]
table = ''.maketrans(before, after)
return s.translate(table)
plaintext = "Python"
key = 3
ciphertext = caesar_cipher_encrypt(plaintext, key)
print("加密后的字符串为:", ciphertext)
```
运行结果为:
```
加密后的字符串为: Sbwkrq
```
阅读全文
相关推荐
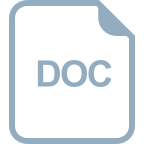
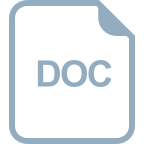
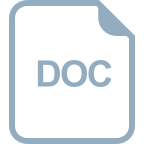
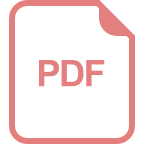
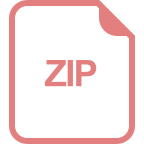
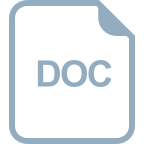





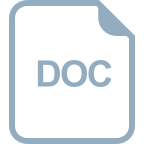
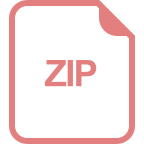
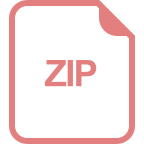
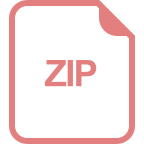
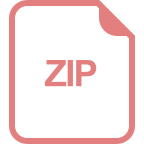
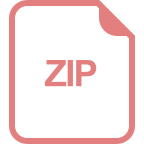
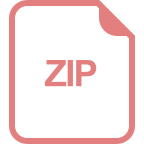