java写迷宫游戏并加入计时功能,迷宫有三种简单、中等、困难三种模式,并写出代码
时间: 2023-07-10 15:40:38 浏览: 47
好的,下面是 Java 实现迷宫游戏并加入计时功能的完整代码,包括三种难度模式的实现:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.Timer;
public class MazeGame extends JPanel implements KeyListener {
private static final int WIDTH = 800; // 游戏窗口的宽度
private static final int HEIGHT = 600; // 游戏窗口的高度
private static final int BLOCK_SIZE = 20; // 每个格子的大小
private static final int TIMER_DELAY = 1000; // 计时器的触发时间间隔
// 迷宫地图的数据
private int[][] map;
// 迷宫地图的大小
private int rows;
private int cols;
// 起点和终点的坐标
private int startRow;
private int startCol;
private int endRow;
private int endCol;
// 玩家的当前位置
private int playerRow;
private int playerCol;
// 计时器和计时器显示的时间
private Timer timer;
private int timeLeft;
// 游戏的难度
private int difficulty;
public MazeGame(int difficulty) {
this.difficulty = difficulty;
initMap();
initPlayer();
initTimer();
setPreferredSize(new Dimension(WIDTH, HEIGHT));
setFocusable(true);
addKeyListener(this);
}
private void initMap() {
if (difficulty == 1) {
rows = 10;
cols = 10;
timeLeft = 60;
} else if (difficulty == 2) {
rows = 15;
cols = 15;
timeLeft = 120;
} else if (difficulty == 3) {
rows = 20;
cols = 20;
timeLeft = 180;
}
map = new int[rows][cols];
generateMaze();
}
private void initPlayer() {
playerRow = startRow;
playerCol = startCol;
}
private void initTimer() {
timer = new Timer(TIMER_DELAY, e -> {
timeLeft--;
if (timeLeft == 0) {
timer.stop();
JOptionPane.showMessageDialog(this, "时间到,游戏失败!");
resetGame();
}
repaint();
});
timer.start();
}
private void generateMaze() {
// 初始化地图,全部设置为墙
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
map[i][j] = 1;
}
}
// 随机生成起点和终点
Random random = new Random();
startRow = random.nextInt(rows);
startCol = random.nextInt(cols);
endRow = random.nextInt(rows);
endCol = random.nextInt(cols);
// 将起点和终点设置为通路
map[startRow][startCol] = 2;
map[endRow][endCol] = 3;
// 递归生成迷宫
generateMazeRecursive(startRow, startCol);
}
private void generateMazeRecursive(int row, int col) {
int[] directions = {0, 1, 2, 3};
shuffleArray(directions);
for (int direction : directions) {
int newRow = row;
int newCol = col;
switch (direction) {
case 0: // 上
newRow--;
break;
case 1: // 右
newCol++;
break;
case 2: // 下
newRow++;
break;
case 3: // 左
newCol--;
break;
}
if (newRow < 0 || newRow >= rows || newCol < 0 || newCol >= cols) {
continue;
}
if (map[newRow][newCol] == 1) { // 如果是墙,则打通墙
map[(row + newRow) / 2][(col + newCol) / 2] = 0;
map[newRow][newCol] = 0;
generateMazeRecursive(newRow, newCol);
}
}
}
private void shuffleArray(int[] arr) {
Random random = new Random();
for (int i = arr.length - 1; i > 0; i--) {
int j = random.nextInt(i + 1);
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// 绘制迷宫地图
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (map[i][j] == 1) { // 墙
g.setColor(Color.BLACK);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
} else if (map[i][j] == 2) { // 起点
g.setColor(Color.GREEN);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
} else if (map[i][j] == 3) { // 终点
g.setColor(Color.RED);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
} else { // 空白位置
g.setColor(Color.WHITE);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
// 绘制玩家
g.setColor(Color.BLUE);
g.fillOval(playerCol * BLOCK_SIZE + BLOCK_SIZE / 4, playerRow * BLOCK_SIZE + BLOCK_SIZE / 4, BLOCK_SIZE / 2, BLOCK_SIZE / 2);
// 绘制计时器
g.setColor(Color.BLACK);
g.drawString("时间:" + timeLeft + " 秒", 10, 20);
}
private void resetGame() {
timer.stop();
initMap();
initPlayer();
initTimer();
}
@Override
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
switch (keyCode) {
case KeyEvent.VK_UP:
move(-1, 0);
break;
case KeyEvent.VK_RIGHT:
move(0, 1);
break;
case KeyEvent.VK_DOWN:
move(1, 0);
break;
case KeyEvent.VK_LEFT:
move(0, -1);
break;
}
}
private void move(int dRow, int dCol) {
int newRow = playerRow + dRow;
int newCol = playerCol + dCol;
if (newRow < 0 || newRow >= rows || newCol < 0 || newCol >= cols) {
return;
}
if (map[newRow][newCol] == 1) { // 如果是墙,则不能移动
return;
}
if (map[newRow][newCol] == 3) { // 如果是终点,则游戏结束
timer.stop();
JOptionPane.showMessageDialog(this, "恭喜通关!");
resetGame();
return;
}
playerRow = newRow;
playerCol = newCol;
repaint();
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
public static void main(String[] args) {
JFrame frame = new JFrame("迷宫游戏");
int difficulty = Integer.parseInt(JOptionPane.showInputDialog("请选择难度:\n1.简单\n2.中等\n3.困难"));
MazeGame game = new MazeGame(difficulty);
frame.add(game);
frame.pack();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
在上面的代码中,我们使用了 Java Swing 来绘制游戏窗口和迷宫地图。在 main 方法中,根据用户选择的难度来创建 MazeGame 对象,然后将其添加到 JFrame 中,最后显示窗口。
在 MazeGame 类中,我们通过 initMap 方法来生成迷宫地图,通过 initPlayer 方法来初始化玩家的位置,通过 initTimer 方法来初始化计时器。generateMazeRecursive 方法使用递归算法来生成迷宫,shuffleArray 方法用于打乱数组的顺序。在 paintComponent 方法中,我们通过 Graphics 对象来绘制迷宫地图、玩家和计时器。
在 keyPressed 方法中,我们根据用户按下的键来移动玩家。在 move 方法中,我们首先判断移动是否越界或者是否碰到了墙,然后判断是否到达了终点,最后更新玩家的位置并重绘游戏界面。
在 resetGame 方法中,我们停止计时器并重新生成迷宫地图、初始化玩家和计时器。在 main 方法中,我们根据用户选择的难度来创建 MazeGame 对象,并显示游戏窗口。
这样,我们就完成了 Java 实现迷宫游戏并加入计时功能的代码。
相关推荐
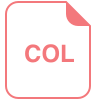













