请帮我编写扫雷静态游戏
时间: 2023-08-12 15:09:26 浏览: 85
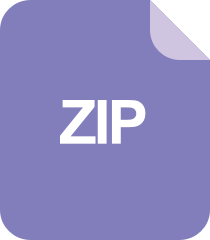
扫雷小游戏,和window上自带的一模一样。用java编写,可以在各大系统运行.zip
好的,下面是一个简单的扫雷静态游戏的 HTML、CSS 和 JavaScript 代码实现:
```html
<!DOCTYPE html>
<html>
<head>
<title>扫雷</title>
<style>
.container {
display: flex;
flex-wrap: wrap;
width: 340px;
margin: 0 auto;
padding: 10px;
background-color: #ccc;
}
.cell {
width: 30px;
height: 30px;
margin: 1px;
background-color: #eee;
text-align: center;
line-height: 30px;
font-size: 20px;
font-weight: bold;
cursor: pointer;
}
.cell:hover {
background-color: #ddd;
}
.cell.bomb {
background-color: #f00;
}
.cell.opened {
background-color: #fff;
border: 1px solid #333;
}
.cell.opened.bomb {
background-color: #f00;
}
.cell.flagged {
background-color: #ffd700;
}
</style>
</head>
<body>
<div class="container"></div>
<script>
// 游戏设置
const ROWS = 10; // 行数
const COLS = 10; // 列数
const BOMBS = 10; // 炸弹数
let board = []; // 游戏面板
let bombs = []; // 炸弹位置
// 初始化游戏面板
function initBoard() {
for (let i = 0; i < ROWS; i++) {
let row = [];
for (let j = 0; j < COLS; j++) {
row.push({
bomb: false,
opened: false,
flagged: false,
count: 0
});
}
board.push(row);
}
}
// 随机生成炸弹位置
function generateBombs() {
while (bombs.length < BOMBS) {
let row = Math.floor(Math.random() * ROWS);
let col = Math.floor(Math.random() * COLS);
if (!board[row][col].bomb) {
board[row][col].bomb = true;
bombs.push({row, col});
}
}
}
// 计算每个格子周围的炸弹数
function calculateCounts() {
for (let i = 0; i < ROWS; i++) {
for (let j = 0; j < COLS; j++) {
if (!board[i][j].bomb) {
let count = 0;
for (let r = i - 1; r <= i + 1; r++) {
for (let c = j - 1; c <= j + 1; c++) {
if (r >= 0 && r < ROWS && c >= 0 && c < COLS && board[r][c].bomb) {
count++;
}
}
}
board[i][j].count = count;
}
}
}
}
// 渲染游戏面板
function renderBoard() {
let container = document.querySelector('.container');
container.innerHTML = '';
for (let i = 0; i < ROWS; i++) {
for (let j = 0; j < COLS; j++) {
let cell = document.createElement('div');
cell.classList.add('cell');
if (board[i][j].opened) {
cell.classList.add('opened');
if (board[i][j].bomb) {
cell.classList.add('bomb');
} else {
cell.innerText = board[i][j].count || '';
}
} else if (board[i][j].flagged) {
cell.classList.add('flagged');
cell.innerText = '🚩';
}
cell.addEventListener('click', () => {
if (!board[i][j].opened && !board[i][j].flagged) {
openCell(i, j);
}
});
cell.addEventListener('contextmenu', (event) => {
event.preventDefault();
if (!board[i][j].opened) {
board[i][j].flagged = !board[i][j].flagged;
renderBoard();
}
});
container.appendChild(cell);
}
}
}
// 打开格子
function openCell(row, col) {
board[row][col].opened = true;
if (board[row][col].bomb) {
gameOver();
} else if (board[row][col].count === 0) {
openEmptyCells(row, col);
} else {
checkWin();
}
renderBoard();
}
// 打开周围的空格子
function openEmptyCells(row, col) {
for (let r = row - 1; r <= row + 1; r++) {
for (let c = col - 1; c <= col + 1; c++) {
if (r >= 0 && r < ROWS && c >= 0 && c < COLS && !board[r][c].opened && !board[r][c].flagged) {
openCell(r, c);
}
}
}
}
// 游戏结束
function gameOver() {
for (let i = 0; i < ROWS; i++) {
for (let j = 0; j < COLS; j++) {
board[i][j].opened = true;
}
}
renderBoard();
alert('游戏结束!');
}
// 检查是否胜利
function checkWin() {
let openedCount = 0;
for (let i = 0; i < ROWS; i++) {
for (let j = 0; j < COLS; j++) {
if (board[i][j].opened) {
openedCount++;
}
}
}
if (openedCount === ROWS * COLS - BOMBS) {
alert('恭喜你获胜!');
}
}
// 开始游戏
function startGame() {
initBoard();
generateBombs();
calculateCounts();
renderBoard();
}
startGame();
</script>
</body>
</html>
```
这份代码实现了一个简单的扫雷静态游戏,代码中包含了游戏的初始化、炸弹位置生成、炸弹周围数字计算、游戏面板渲染、格子打开和游戏结束等功能。你可以根据自己的需要修改和优化这份代码。
阅读全文
相关推荐
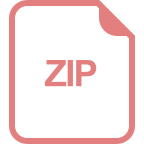
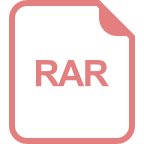
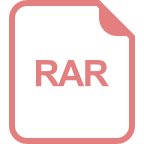
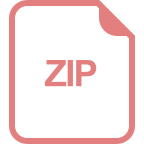
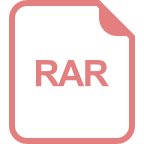
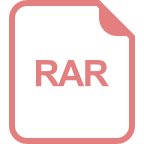
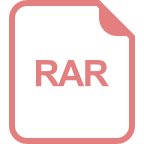
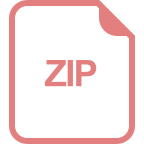
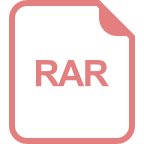
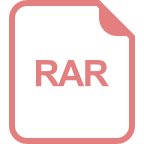
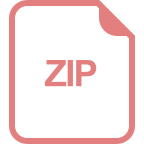
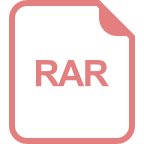
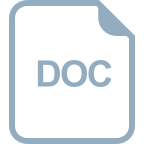
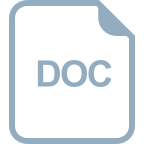
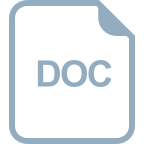
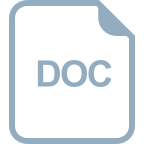
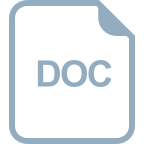