直接插入排序C++
时间: 2023-07-02 13:12:24 浏览: 88
以下是直接插入排序的C++代码实现:
```c++
void insertionSort(int arr[], int n) {
int i, key, j;
for (i = 1; i < n; i++) {
key = arr[i];
j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
```
这个算法的时间复杂度为O(n^2),适用于小规模数据的排序。
相关问题
直接插入排序c++
以下是插入排序的 C++ 实现代码:
```cpp
void insertionSort(int arr[], int n) {
int i, key, j;
for (i = 1; i < n; i++) {
key = arr[i];
j = i - 1;
/* Move elements of arr[0..i-1], that are greater than key, to one position ahead of their current position */
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
```
其中 `arr[]` 表示待排序的数组,`n` 表示数组的长度。该算法会对数组进行升序排序。
数据结构直接插入排序C++
数据结构中的直接插入排序是一种简单的排序算法,它的工作原理类似于打扑克牌时的整理过程。在C++中,它的基本步骤如下:
1. **初始化**:假设有一个未排序的数组,开始时第一个元素被视为已排序。
2. **遍历**:从第二个元素开始,对于每个元素(`i`),将其视为待插入的元素。
3. **比较和插入**:从当前元素的前一个位置(`j = i - 1`)开始,如果`arr[j]`大于待插入的元素,则将`arr[j]`向右移动一位。这个过程一直持续到找到一个比待插入元素小的位置,或者到达数组的第一个元素。
4. **插入**:然后将待插入的元素放置在找到的合适位置,并将后续元素相应地向前移动。
5. **重复**:继续处理下一个元素,直到数组完全排序。
以下是C++中直接插入排序的简单示例:
```cpp
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; ++i) {
int key = arr[i];
int j = i - 1;
// 将arr[j]向右移位,直到找到一个小于key的位置
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key; // 插入key到正确位置
}
}
```
阅读全文
相关推荐





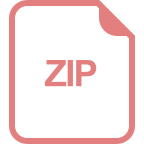






