java web企业门户网站的公告代码
时间: 2023-09-14 15:05:09 浏览: 42
以下是一个简单的 Java Web 企业门户网站公告的代码示例:
1. 公告实体类
```java
public class Notice {
private int id;
private String title;
private String content;
private Date createTime;
// getter 和 setter 方法
}
```
2. 公告 DAO 接口
```java
public interface NoticeDao {
// 添加公告
public boolean addNotice(Notice notice) throws SQLException;
// 删除公告
public boolean deleteNotice(int noticeId) throws SQLException;
// 修改公告
public boolean updateNotice(Notice notice) throws SQLException;
// 查询所有公告
public List<Notice> findAllNotices() throws SQLException;
// 根据 ID 查询公告
public Notice findNoticeById(int noticeId) throws SQLException;
}
```
3. 公告 DAO 实现类
```java
public class NoticeDaoImpl extends BaseDao implements NoticeDao {
@Override
public boolean addNotice(Notice notice) throws SQLException {
String sql = "INSERT INTO notice(title,content,create_time) VALUES(?,?,?)";
Object[] params = {notice.getTitle(), notice.getContent(), notice.getCreateTime()};
return executeUpdate(sql, params);
}
@Override
public boolean deleteNotice(int noticeId) throws SQLException {
String sql = "DELETE FROM notice WHERE id=?";
Object[] params = {noticeId};
return executeUpdate(sql, params);
}
@Override
public boolean updateNotice(Notice notice) throws SQLException {
String sql = "UPDATE notice SET title=?,content=?,create_time=? WHERE id=?";
Object[] params = {notice.getTitle(), notice.getContent(), notice.getCreateTime(), notice.getId()};
return executeUpdate(sql, params);
}
@Override
public List<Notice> findAllNotices() throws SQLException {
String sql = "SELECT * FROM notice";
ResultSet rs = executeQuery(sql, null);
List<Notice> noticeList = new ArrayList<>();
while (rs.next()) {
Notice notice = new Notice();
notice.setId(rs.getInt("id"));
notice.setTitle(rs.getString("title"));
notice.setContent(rs.getString("content"));
notice.setCreateTime(rs.getDate("create_time"));
noticeList.add(notice);
}
return noticeList;
}
@Override
public Notice findNoticeById(int noticeId) throws SQLException {
String sql = "SELECT * FROM notice WHERE id=?";
Object[] params = {noticeId};
ResultSet rs = executeQuery(sql, params);
Notice notice = null;
if (rs.next()) {
notice = new Notice();
notice.setId(rs.getInt("id"));
notice.setTitle(rs.getString("title"));
notice.setContent(rs.getString("content"));
notice.setCreateTime(rs.getDate("create_time"));
}
return notice;
}
}
```
4. 公告服务类
```java
public class NoticeService {
private NoticeDao noticeDao = new NoticeDaoImpl();
// 添加公告
public boolean addNotice(Notice notice) {
try {
return noticeDao.addNotice(notice);
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
// 删除公告
public boolean deleteNotice(int noticeId) {
try {
return noticeDao.deleteNotice(noticeId);
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
// 修改公告
public boolean updateNotice(Notice notice) {
try {
return noticeDao.updateNotice(notice);
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
// 查询所有公告
public List<Notice> findAllNotices() {
try {
return noticeDao.findAllNotices();
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
// 根据 ID 查询公告
public Notice findNoticeById(int noticeId) {
try {
return noticeDao.findNoticeById(noticeId);
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
}
```
5. 公告控制器
```java
@WebServlet("/notice/*")
public class NoticeServlet extends HttpServlet {
private NoticeService noticeService = new NoticeService();
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String pathInfo = request.getPathInfo();
if ("/list".equals(pathInfo)) {
list(request, response);
} else if ("/detail".equals(pathInfo)) {
detail(request, response);
}
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String pathInfo = request.getPathInfo();
if ("/add".equals(pathInfo)) {
add(request, response);
} else if ("/delete".equals(pathInfo)) {
delete(request, response);
} else if ("/update".equals(pathInfo)) {
update(request, response);
}
}
// 添加公告
private void add(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String title = request.getParameter("title");
String content = request.getParameter("content");
Date createTime = new Date();
Notice notice = new Notice();
notice.setTitle(title);
notice.setContent(content);
notice.setCreateTime(createTime);
boolean result = noticeService.addNotice(notice);
if (result) {
response.sendRedirect(request.getContextPath() + "/notice/list");
} else {
request.setAttribute("message", "添加公告失败");
request.getRequestDispatcher("/WEB-INF/views/notice/add.jsp").forward(request, response);
}
}
// 删除公告
private void delete(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int noticeId = Integer.parseInt(request.getParameter("id"));
boolean result = noticeService.deleteNotice(noticeId);
if (result) {
response.sendRedirect(request.getContextPath() + "/notice/list");
} else {
request.setAttribute("message", "删除公告失败");
request.getRequestDispatcher("/WEB-INF/views/notice/list.jsp").forward(request, response);
}
}
// 修改公告
private void update(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int noticeId = Integer.parseInt(request.getParameter("id"));
String title = request.getParameter("title");
String content = request.getParameter("content");
Date createTime = new Date();
Notice notice = new Notice();
notice.setId(noticeId);
notice.setTitle(title);
notice.setContent(content);
notice.setCreateTime(createTime);
boolean result = noticeService.updateNotice(notice);
if (result) {
response.sendRedirect(request.getContextPath() + "/notice/list");
} else {
request.setAttribute("message", "修改公告失败");
request.getRequestDispatcher("/WEB-INF/views/notice/edit.jsp").forward(request, response);
}
}
// 查询所有公告
private void list(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Notice> noticeList = noticeService.findAllNotices();
request.setAttribute("noticeList", noticeList);
request.getRequestDispatcher("/WEB-INF/views/notice/list.jsp").forward(request, response);
}
// 根据 ID 查询公告
private void detail(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int noticeId = Integer.parseInt(request.getParameter("id"));
Notice notice = noticeService.findNoticeById(noticeId);
request.setAttribute("notice", notice);
request.getRequestDispatcher("/WEB-INF/views/notice/detail.jsp").forward(request, response);
}
}
```
以上代码仅供参考,具体实现可能会根据实际需求有所调整。
相关推荐
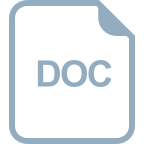












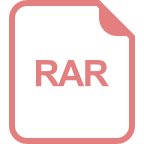
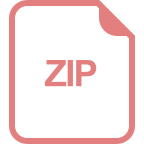
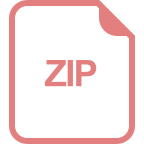