用c语言编写一个简单的shell,其中main包含内部指令cd,clr,dir,environ,help,pause,quit。
时间: 2023-05-21 15:03:24 浏览: 58
好的,以下是一个简单的C语言shell程序,其中包含了内部指令cd,clr,dir,environ,help,pause和quit:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
#define MAX_COMMAND_LENGTH 100
#define MAX_NUM_ARGUMENTS 10
int main()
{
char *command;
char *arguments[MAX_NUM_ARGUMENTS];
char input[MAX_COMMAND_LENGTH];
int status;
while (1)
{
printf("shell> ");
fgets(input, MAX_COMMAND_LENGTH, stdin);
// Remove newline character from input
input[strlen(input) - 1] = '\0';
// Parse command and arguments
command = strtok(input, " ");
int i = 0;
while (command != NULL && i < MAX_NUM_ARGUMENTS - 1)
{
arguments[i] = command;
command = strtok(NULL, " ");
i++;
}
arguments[i] = NULL;
// Handle internal commands
if (strcmp(arguments[0], "cd") == 0)
{
if (arguments[1] == NULL)
{
printf("cd: missing argument\n");
}
else if (chdir(arguments[1]) != 0)
{
printf("cd: %s: No such file or directory\n", arguments[1]);
}
}
else if (strcmp(arguments[0], "clr") == 0)
{
system("clear");
}
else if (strcmp(arguments[0], "dir") == 0)
{
pid_t pid = fork();
if (pid == 0)
{
execlp("ls", "ls", "-l", NULL);
exit(0);
}
else
{
waitpid(pid, &status, 0);
}
}
else if (strcmp(arguments[0], "environ") == 0)
{
char **env = environ;
while (*env != NULL)
{
printf("%s\n", *env);
env++;
}
}
else if (strcmp(arguments[0], "help") == 0)
{
printf("cd <directory> Change the current default directory to <directory>\n");
printf("clr Clear the screen\n");
printf("dir List the contents of the current directory in long format\n");
printf("environ List all the environment strings\n");
printf("help Display this help message\n");
printf("pause Pause operation of the shell until 'Enter' is pressed\n");
printf("quit Quit the shell\n");
}
else if (strcmp(arguments[0], "pause") == 0)
{
printf("Press Enter to continue...");
getchar();
}
else if (strcmp(arguments[0], "quit") == 0)
{
exit(0);
}
else
{
// Handle external commands
pid_t pid = fork();
if (pid == 0)
{
execvp(arguments[0], arguments);
printf("%s: command not found\n", arguments[0]);
exit(0);
}
else
{
waitpid(pid, &status, 0);
}
}
}
return 0;
}
```
希望这个程序能够满足您的需求!
相关推荐
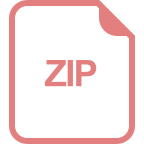
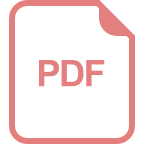
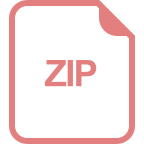














