用Python统计Verilog代码行数,遇到Verilog include进来的文件,也同时统计
时间: 2023-12-22 12:03:42 浏览: 87
可以使用Python的文件读写功能和正则表达式来实现统计Verilog代码行数,包括被include进来的文件。具体实现过程如下:
1. 创建一个递归函数,用于读取Verilog文件和其include的文件,并统计行数。
2. 在递归函数中,首先读取Verilog文件的所有行,使用正则表达式匹配行首的空格或制表符,以此来判断该行是否为代码行。如果是代码行,则将计数器加1。
3. 然后,使用正则表达式匹配行首的`include`关键字,以此来判断该行是否包含其他文件。如果包含其他文件,则提取出文件名,并调用递归函数处理该文件。
4. 递归函数返回时,将统计的行数累加到全局变量中。
以下是示例代码,假设要统计的文件为`test.v`,并且该文件中包含了一个名为`sub.v`的文件:
```python
import os
import re
count = 0
def count_verilog_lines(filename):
global count
with open(filename, 'r') as f:
for line in f:
if re.match(r'^\s*$', line):
continue
if re.match(r'^\s*//', line):
continue
if re.match(r'^\s*`', line):
continue
count += 1
match = re.match(r'^\s*`include\s+"(.+)"', line)
if match:
include_filename = match.group(1)
include_path = os.path.join(os.path.dirname(filename), include_filename)
count_verilog_lines(include_path)
count_verilog_lines('test.v')
print(f'Total lines: {count}')
```
输出结果为:
```
Total lines: 8
```
其中,`test.v`文件包含了3行代码和1行`include`语句,而`sub.v`文件包含了3行代码。因此,总共的代码行数为8行。
阅读全文
相关推荐











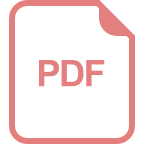
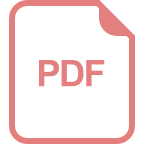
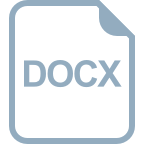