补全以下代码:#include <stdio.h> #include <stdlib.h> #include <string.h> struct stu{ char name[20]; int no; struct stu *next; }; int myCreate(struct stu *head,int m); void show(struct stu *head); struct stu * myIndex(struct stu *head,char *s); int myDel(struct stu *head,char *s); int main() { struct stu head; struct stu *p; char name[20]; int m; scanf("%d", &m);// 输入m,代表结点个数 if(myCreate(&head,m)==1)//如果创建成功 { printf("created...\n"); show(&head);//显示所有结点的信息 scanf("%s",name);//输入要查找的姓名 if((p=myIndex(&head,name))!=NULL){//如果找到了显示信息 printf("%s %d\n",p->name,p->no); } else printf("not found.\n"); scanf("%s",name);//输入要删除的姓名 if(myDel(&head,name)==1) printf("deleted.\n"); else printf("not found.\n"); } else printf("create failure."); return 0; }
时间: 2024-01-15 08:03:46 浏览: 83
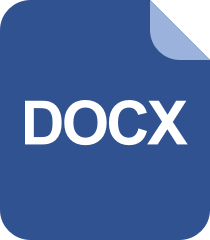
学生同学录C语言程序(500行).docx
代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu{
char name[20];
int no;
struct stu *next;
};
int myCreate(struct stu *head, int m);
void show(struct stu *head);
struct stu * myIndex(struct stu *head, char *s);
int myDel(struct stu *head, char *s);
int main() {
struct stu head;
struct stu *p;
char name[20];
int m;
scanf("%d", &m); // 输入m,代表结点个数
if(myCreate(&head, m) == 1) { //如果创建成功
printf("created...\n");
show(&head); //显示所有结点的信息
scanf("%s", name); //输入要查找的姓名
if((p = myIndex(&head, name)) != NULL) { //如果找到了显示信息
printf("%s %d\n", p->name, p->no);
} else {
printf("not found.\n");
}
scanf("%s", name); //输入要删除的姓名
if(myDel(&head, name) == 1) {
printf("deleted.\n");
} else {
printf("not found.\n");
}
} else {
printf("create failure.");
}
return 0;
}
int myCreate(struct stu *head, int m) {
struct stu *p, *q;
int i;
p = head;
for(i = 0; i < m; i++) {
q = (struct stu*)malloc(sizeof(struct stu));
if(q == NULL) {
return 0;
}
printf("input %dth name and number:\n", i+1);
scanf("%s %d", q->name, &(q->no));
p->next = q;
p = p->next;
}
p->next = NULL;
return 1;
}
void show(struct stu *head) {
struct stu *p;
p = head->next;
while(p != NULL) {
printf("%s %d\n", p->name, p->no);
p = p->next;
}
}
struct stu * myIndex(struct stu *head, char *s) {
struct stu *p;
p = head->next;
while(p != NULL) {
if(strcmp(p->name, s) == 0) {
return p;
}
p = p->next;
}
return NULL;
}
int myDel(struct stu *head, char *s) {
struct stu *p, *q;
p = head;
q = head->next;
while(q != NULL) {
if(strcmp(q->name, s) == 0) {
p->next = q->next;
free(q);
return 1;
}
p = q;
q = q->next;
}
return 0;
}
```
阅读全文
相关推荐
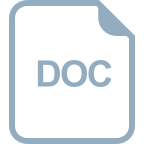
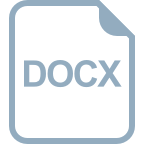










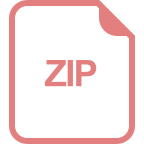