修改如下代码:#include<stdio.h> #include<stdlib.h> struct stu { char name[20]; int no; struct stu *next; }; int create(struct stu *head,int n); void research(struct stu *head); int main() { int a,i; struct stu *head; head->next=NULL; scanf("%d",&a); create(head,a); research(head); return 0; } int create(struct stu *head,int n) { int i; head->next=NULL; struct stu *node; for(i=0;i<n;i++) { node= (struct stu*)malloc(sizeof(node)) ; scanf("%d",&node->no); node->next=head->next; head->next=node; } return 1; } void research(struct stu *head) { struct stu *p; p=head->next; while(p!=NULL) { printf("%d\n",p->no); p=p->next; } }
时间: 2024-03-22 08:41:03 浏览: 21
修改后的代码如下:
```c
#include<stdio.h>
#include<stdlib.h>
struct stu {
char name[20];
int no;
struct stu *next;
};
int create(struct stu *head, int n);
void research(struct stu *head);
int main() {
int a,i;
struct stu *head = (struct stu*)malloc(sizeof(struct stu)); // 为头结点分配内存
head->next = NULL;
scanf("%d",&a);
create(head, a);
research(head);
return 0;
}
int create(struct stu *head, int n) {
int i;
struct stu *node;
for(i=0; i<n; i++) {
node = (struct stu*)malloc(sizeof(struct stu));
scanf("%d",&node->no);
node->next = head->next;
head->next = node;
}
return 1;
}
void research(struct stu *head) {
struct stu *p;
p = head->next;
while(p != NULL) {
printf("%d\n",p->no);
p = p->next;
}
}
```
主要的修改如下:
1. 在`main`函数中为头结点动态分配内存,并将`head->next`初始化为`NULL`。
2. 在`create`函数中为每个新结点动态分配内存。
3. 在函数参数中将`*head`改为`head`,因为在函数内部并不会修改头指针的值,只需要修改头指针所指向的结点即可。
相关推荐
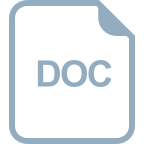
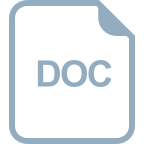
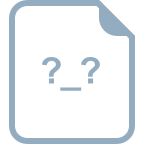










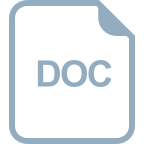
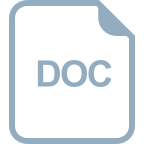
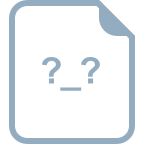
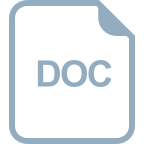
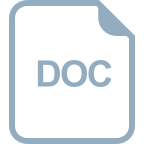