hutool-http使用示例
时间: 2023-07-11 08:47:49 浏览: 208
好的,以下是一个简单的hutool-http使用示例:
```java
import cn.hutool.http.HttpUtil;
public class HttpDemo {
public static void main(String[] args) {
// 发送GET请求
String result = HttpUtil.get("https://www.baidu.com");
System.out.println(result);
// 发送POST请求
String url = "http://localhost:8080/login";
String paramJson = "{\"username\":\"admin\",\"password\":\"123456\"}";
String result2 = HttpUtil.post(url, paramJson);
System.out.println(result2);
}
}
```
在这个示例中,我们使用HttpUtil类的get和post方法分别发送了GET和POST请求。其中,get方法发送了一个请求到百度首页,获取了页面内容并打印输出。post方法发送了一个登录请求到本地服务器,并将结果打印输出。这只是一个简单的示例,hutool-http还支持更多的请求方式和参数配置,您可以根据自己的需求进行使用和配置。
相关问题
curl 转换hutool Hutool-http
使用Hutool的HttpUtil可以实现类似curl的功能,以下是一个简单的示例代码:
```java
import cn.hutool.http.HttpUtil;
public class Test {
public static void main(String[] args) {
// 设置请求头
String userAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3";
String referer = "https://www.baidu.com/";
String accept = "text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8";
String charset = "UTF-8";
String contentType = "application/x-www-form-urlencoded";
HttpUtil.createGet("http://www.hutool.cn")
.header("User-Agent", userAgent)
.header("Referer", referer)
.header("Accept", accept)
.charset(charset)
.contentType(contentType)
// 设置请求参数
.form("key1", "value1")
.form("key2", "value2")
.execute();
}
}
```
以上代码实现了一个GET请求,设置了请求头和请求参数。如果需要发送POST请求,只需要将`HttpUtil.createGet()`改为`HttpUtil.createPost()`,并使用`body()`方法设置请求体即可。
注意:Hutool-http需要引入Hutool-all依赖。
使用hutool的SoapClient的示例
Hutool的SoapClient是一个用于处理SOAP协议的工具类,它可以帮助开发者方便地发送SOAP请求并解析响应结果。以下是一个简单的Hutool SoapClient的使用示例:
```java
import cn.hutool.core.io.IoUtil;
import cn.hutool.http.HttpMethod;
import cn.hutool.http.soap.SoapClient;
public class SoapExample {
public static void main(String[] args) {
// 创建一个SoapClient实例,指定基础URL
SoapClient soap = new SoapClient("http://example.com/api?wsdl");
// 定义要发送的SOAP消息体,这里以XML字符串形式表示
String xmlMessage = "<soapenv:Envelope xmlns:soapenv='http://schemas.xmlsoap.org/soap/envelope/'><soapenv:Body><sayHelloRequest><name>John Doe</name></sayHelloRequest></soapenv:Body></soapenv:Envelope>";
try {
// 发送POST请求,并附带SOAP消息体
String response = soap.post(xmlMessage, SoapClient.ContentType.XML);
// 解析响应
String result = IoUtil.readToString(response);
System.out.println("Response: " + result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个例子中,首先创建了一个`SoapClient`对象,并指定了服务的WSDL地址。然后定义了SOAP请求的消息体,通过`post`方法发送请求,接收服务器返回的数据。最后,将响应内容打印出来。
阅读全文
相关推荐
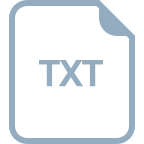
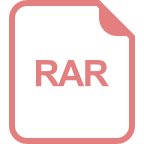
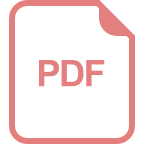
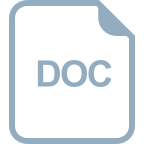
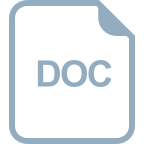











