#include <stdio.h> #define N 10 int fun ( int *a, int n ) { int max=a[0],secondmax=a[0],temp,temp1; for(int j=0;j<n;j++) { if(a[j]>max) { max=a[j]; } else if(a[j]>secondmax) { secondmax=a[j]; } } max=temp; max=a[0]; temp=a[0]; secondmax=temp1; secondmax=a[1]; temp1=a[0]; } int main( ) { int x, b[N]={11,5,12,0,3,6,9,7,10,8}, n=10, i; for ( i=0; i<n; i++ ) printf("%d ", b[i]); printf("\n"); fun ( b, n ); for ( i=0; i<n; i++ ) printf("%d ", b[i]); printf("\n"); return 0; }帮我找出问题
时间: 2024-01-14 19:04:05 浏览: 43
这段代码中有几个问题:
1. 函数 `fun` 的返回值类型是 `int`,但是没有返回任何值,需要在函数末尾添加 `return` 语句。
2. 在函数 `fun` 中,变量 `temp` 和 `temp1` 没有被正确赋值。应该将 `temp=a[0];` 和 `temp1=a[1];` 放在函数开头。
3. 在函数 `fun` 中,变量 `max` 和 `secondmax` 的赋值有误。应该将 `max=temp;` 和 `secondmax=temp1;` 改为 `temp=max;` 和 `temp1=secondmax;`。
4. 在 `main` 函数中,变量 `x` 没有被使用,可以删除。
修改后的代码如下:
```c
#include <stdio.h>
#define N 10
int fun(int *a, int n) {
int max = a[0], secondmax = a[0], temp = a[0], temp1 = a[1];
for (int j = 0; j < n; j++) {
if (a[j] > max) {
secondmax = max;
max = a[j];
}
else if (a[j] > secondmax) {
secondmax = a[j];
}
}
a[0] = max;
a[1] = secondmax;
return 0;
}
int main() {
int b[N] = {11, 5, 12, 0, 3, 6, 9, 7, 10, 8}, n = 10, i;
for (i = 0; i < n; i++) {
printf("%d ", b[i]);
}
printf("\n");
fun(b, n);
for (i = 0; i < n; i++) {
printf("%d ", b[i]);
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
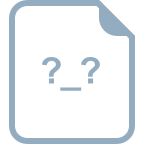
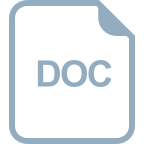
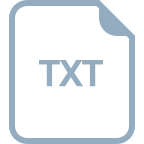















