pyqt5类的函数中创建线程,线程要用类中的变量,怎么写这个代码
时间: 2023-07-15 14:13:00 浏览: 145
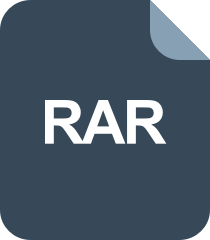
类成员函数创建线程源码

可以使用Python的`threading`模块来创建线程,并通过信号与槽机制(Signal-Slot mechanism)来访问类中的变量。
下面是一个简单的例子:
```python
import sys
import threading
from PyQt5.QtCore import QObject, pyqtSignal
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QLabel
class MyThread(threading.Thread):
def __init__(self, signal, parent=None):
super(MyThread, self).__init__(parent)
self.signal = signal
def run(self):
for i in range(10):
self.signal.emit(str(i))
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setGeometry(100, 100, 250, 200)
self.label = QLabel('Waiting for thread...', self)
self.label.move(50, 50)
self.button = QPushButton('Start Thread', self)
self.button.move(50, 100)
self.button.clicked.connect(self.start_thread)
self.thread_signal = pyqtSignal(str)
self.thread = MyThread(self.thread_signal)
self.thread_signal.connect(self.update_label)
def start_thread(self):
self.thread.start()
def update_label(self, message):
self.label.setText('Thread says: ' + message)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个例子中,我们定义了一个`MyThread`类,它继承自`threading.Thread`类,重写了`run`方法,在该方法中通过`self.signal.emit`发送信号。同时,在`MainWindow`类中,我们创建了一个`MyThread`对象和一个`pyqtSignal`对象,并将它们通过`self.thread_signal.connect`连接起来。当线程中有数据时,会通过信号发出来,然后在`MainWindow`类中的`update_label`方法中更新界面中的标签文本。
需要注意的是,由于线程中访问了类中的变量,因此在访问时需要考虑线程安全性,可以使用Python的线程锁(`threading.Lock`)来实现。
阅读全文
相关推荐
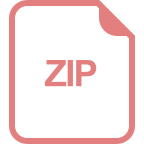
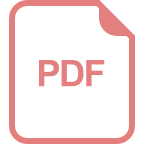
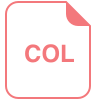



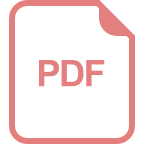
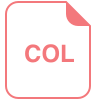
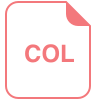







