Java实现复数加减乘除
时间: 2023-07-11 22:50:43 浏览: 127
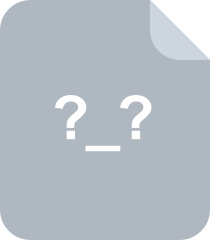
Java中复数类的计算,对复数进行加减乘除
在 Java 中,我们可以定义一个复数类来实现复数的加减乘除运算。下面是一个简单的复数类的实现:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
double real = this.real + other.real;
double imag = this.imag + other.imag;
return new Complex(real, imag);
}
public Complex subtract(Complex other) {
double real = this.real - other.real;
double imag = this.imag - other.imag;
return new Complex(real, imag);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
public double getReal() {
return real;
}
public double getImag() {
return imag;
}
@Override
public String toString() {
return real + " + " + imag + "i";
}
}
```
在上面的代码中,我们定义了一个 Complex 类,它包含实部和虚部两个成员变量。这个类还包含了四个方法:add、subtract、multiply 和 divide,分别用于实现复数的加、减、乘和除运算。这个类还提供了 getter 方法来获取实部和虚部的值,以及一个 toString 方法来将复数转换成字符串形式。
下面是一个使用这个类的例子:
```java
public class Main {
public static void main(String[] args) {
Complex a = new Complex(1, 2);
Complex b = new Complex(3, 4);
Complex sum = a.add(b);
Complex diff = a.subtract(b);
Complex product = a.multiply(b);
Complex quotient = a.divide(b);
System.out.println("a = " + a);
System.out.println("b = " + b);
System.out.println("a + b = " + sum);
System.out.println("a - b = " + diff);
System.out.println("a * b = " + product);
System.out.println("a / b = " + quotient);
}
}
```
在上面的代码中,我们创建了两个复数对象 a 和 b,并分别进行了加、减、乘和除运算,并将结果输出到控制台上。
阅读全文
相关推荐
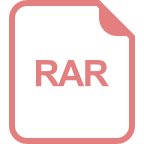















