freemodbus stm32 hal库porttimer代码,中文注释
时间: 2023-08-21 19:02:45 浏览: 147
以下是FreeModbus STM32 HAL库中porttimer文件的示例代码,其中包含了中文注释:
```
/*
* FreeModbus Libary: STM32Fxxx Port Timer For Modbus
*
* Copyright (C) 2013 Real Time Engineers ltd.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*
* File: porttimer.c
*
* Description: STM32Fxxx port timer implementation for FreeModbus.
*/
/* ----------------------- Modbus includes ----------------------------------*/
#include "mb.h"
#include "mbport.h"
/* ----------------------- STM32 includes ----------------------------------*/
#include "stm32f10x.h"
/* ----------------------- Defines ------------------------------------------*/
#define MB_TIMER_PRESCALER ( ( uint16_t )( ( SystemCoreClock / 2 ) / 1000000 ) - 1 ) // 定时器预分频器,用于将系统时钟分频到1MHz
#define MB_TIMER_TICKS ( 20000UL ) // 定时器重装值,用于定时20ms
/* ----------------------- Static variables ---------------------------------*/
static USHORT usTimerOCRADelta;
/* ----------------------- Function prototypes ------------------------------*/
static void prvvTIMERExpiredISR( void );
/* ----------------------- Start implementation -----------------------------*/
BOOL
xMBPortTimersInit( USHORT usTim1Timerout50us )
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
/* Enable the TIM2 global Interrupt */
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 3;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init( &NVIC_InitStructure );
/* Time base configuration */
TIM_TimeBaseStructInit( &TIM_TimeBaseStructure );
TIM_TimeBaseStructure.TIM_Period = ( uint16_t )( MB_TIMER_TICKS - 1 );
TIM_TimeBaseStructure.TIM_Prescaler = MB_TIMER_PRESCALER;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit( TIM2, &TIM_TimeBaseStructure );
/* Clear TIM2 update flag */
TIM_ClearFlag( TIM2, TIM_FLAG_Update );
/* Enable TIM2 Update interrupt */
TIM_ITConfig( TIM2, TIM_IT_Update, ENABLE );
/* Preload enable */
TIM_OC1PreloadConfig( TIM2, TIM_OCPreload_Disable );
TIM_ARRPreloadConfig( TIM2, ENABLE );
/* TIM2 enable counter */
TIM_Cmd( TIM2, ENABLE );
/* Timer delta value for Modbus tick */
usTimerOCRADelta = ( uint16_t )( ( ( uint32_t )usTim1Timerout50us * ( uint32_t )( MB_TIMER_TICKS ) ) / ( uint32_t )20000 );
return TRUE;
}
void
vMBPortTimersEnable( )
{
/* Enable the timer with the timeout passed to xMBPortTimersInit( ) */
TIM_SetAutoreload( TIM2, ( uint16_t )( MB_TIMER_TICKS - 1 ) );
TIM_SetCompare1( TIM2, TIM_GetCounter( TIM2 ) + usTimerOCRADelta );
TIM_Cmd( TIM2, ENABLE );
}
void
vMBPortTimersDisable( )
{
/* Disable any pending timers. */
TIM_Cmd( TIM2, DISABLE );
TIM_SetCounter( TIM2, 0 );
}
/* ----------------------- Timers functions ---------------------------------*/
void
prvvTIMERExpiredISR( void )
{
( void )pxMBPortCBTimerExpired( );
}
void
TIM2_IRQHandler( void )
{
if( TIM_GetITStatus( TIM2, TIM_IT_Update ) != RESET )
{
TIM_ClearITPendingBit( TIM2, TIM_IT_Update );
prvvTIMERExpiredISR( );
}
}
```
这段代码实现了FreeModbus STM32 HAL库的定时器功能。其中,`MB_TIMER_PRESCALER`和`MB_TIMER_TICKS`分别是定时器的预分频器和重装值,用于将定时器时钟分频到1MHz并定时20ms。在`xMBPortTimersInit()`函数中,首先配置了定时器的各项参数,并设置了定时器中断的优先级和使能。在`vMBPortTimersEnable()`函数中,设置了定时器到期时间,并使能了定时器。而在`vMBPortTimersDisable()`函数中,则禁用了定时器。
此外,代码还实现了定时器到期时的中断处理函数`prvvTIMERExpiredISR()`和定时器中断服务函数`TIM2_IRQHandler()`,以便在定时器到期时触发Modbus超时处理。
阅读全文
相关推荐
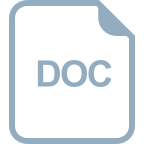
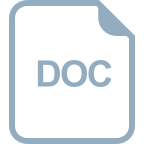
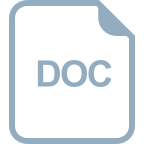
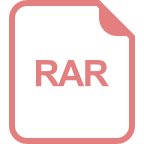

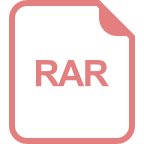
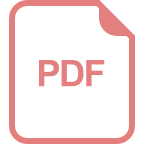

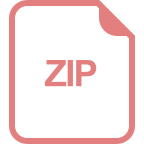
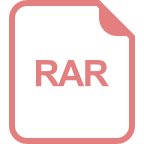
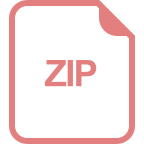
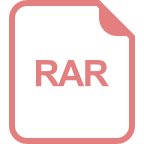
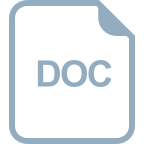




