python购物商品管理使用系统需要登录验证,进入系统后,输入购物预算信息 用户可以任意查看商品信息和已购商品信息 用户根据商品编号购买商品,购买时,检测余额是否够,够就直接付款,不够就提醒 可随时退出,退出后,打印已购买商品和余额
时间: 2024-04-29 11:23:12 浏览: 51
信息。
以下是一个简单的实现:
```python
# 商品信息
products = {
'001': {'name': '手机', 'price': 2000},
'002': {'name': '电脑', 'price': 5000},
'003': {'name': '平板', 'price': 3000},
}
# 已购买商品信息
bought_products = {}
# 登录验证
def login():
# TODO: 实现登录验证逻辑
return True
# 输入购物预算信息
def input_budget():
budget = float(input('请输入您的购物预算:'))
return budget
# 查看商品信息
def view_products():
print('商品编号\t商品名称\t商品价格')
for code, info in products.items():
print(f'{code}\t\t{info["name"]}\t\t{info["price"]}')
# 购买商品
def buy_product(budget):
code = input('请输入要购买的商品编号:')
if code not in products:
print('商品不存在')
return
price = products[code]['price']
if budget < price:
print('余额不足')
return
name = products[code]['name']
bought_products[code] = {'name': name, 'price': price}
budget -= price
print(f'购买成功!已购买商品:{name},花费:{price}元,余额:{budget}元')
return budget
# 打印已购买商品和余额信息
def print_info():
print('已购买商品:')
total_price = 0
for code, info in bought_products.items():
print(f'{info["name"]}\t\t{info["price"]}')
total_price += info['price']
print(f'总计:{total_price}元')
budget = input_budget() # 重新输入预算
print(f'余额:{budget}元')
# 主程序
if login():
budget = input_budget()
while True:
print('请选择操作:')
print('1. 查看商品信息')
print('2. 购买商品')
print('3. 退出')
choice = input()
if choice == '1':
view_products()
elif choice == '2':
budget = buy_product(budget)
elif choice == '3':
print_info()
break
else:
print('无效操作')
```
注意,这只是一个简单的实现,实际使用中还需要考虑很多细节和安全性问题。
阅读全文
相关推荐
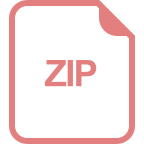
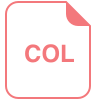
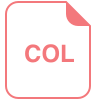
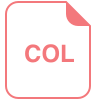
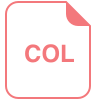
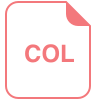
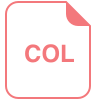
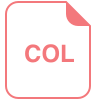
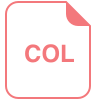
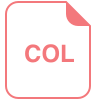
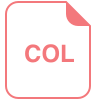
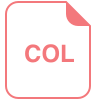
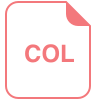
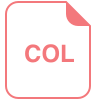
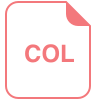
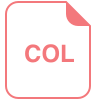