STM32使用LIBJPEG例程
时间: 2023-07-11 12:55:27 浏览: 812
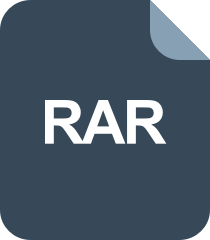
STM32 使用实例
使用LIBJPEG库在STM32上进行JPEG图像的解码和编码是比较常见的应用。下面是一个简单的例程,展示如何在STM32上使用LIBJPEG库进行JPEG图像的解码和编码。
首先,需要将LIBJPEG库添加到STM32项目中。可以从LIBJPEG官网下载最新版本的库文件,并将其添加到STM32项目中。在添加库文件时,需要注意将库文件的头文件和源文件都添加到项目中。
接下来,需要编写代码来初始化JPEG解码器和编码器。例如,以下代码初始化了JPEG解码器:
```
/* JPEG decoding object */
jpeg_decompress_struct cinfo;
/* Error handler object */
jpeg_error_mgr jerr;
/* Initialize the JPEG decompression object with default error handling */
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_decompress(&cinfo);
```
然后,需要读取JPEG图像文件并将其解码。以下代码展示了如何读取并解码JPEG图像:
```
/* Open the input file */
FILE *infile = fopen("input.jpg", "rb");
if (infile == NULL) {
printf("Error opening input file");
return;
}
/* Specify the input source */
jpeg_stdio_src(&cinfo, infile);
/* Read the JPEG header */
jpeg_read_header(&cinfo, TRUE);
/* Start the decompressor */
jpeg_start_decompress(&cinfo);
/* Allocate memory for the image buffer */
unsigned char *buffer = (unsigned char*)malloc(cinfo.output_width * cinfo.output_height * cinfo.output_components);
/* Read the image data */
unsigned char *row_pointer;
while (cinfo.output_scanline < cinfo.output_height) {
row_pointer = buffer + cinfo.output_scanline * cinfo.output_width * cinfo.output_components;
jpeg_read_scanlines(&cinfo, &row_pointer, 1);
}
/* Finish decompression and release resources */
jpeg_finish_decompress(&cinfo);
jpeg_destroy_decompress(&cinfo);
fclose(infile);
```
最后,可以使用LIBJPEG库来编码JPEG图像。以下代码展示了如何使用LIBJPEG库来编码JPEG图像:
```
/* JPEG encoding object */
jpeg_compress_struct cinfo;
/* Error handler object */
jpeg_error_mgr jerr;
/* Initialize the JPEG compression object with default error handling */
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
/* Specify the output file */
FILE *outfile = fopen("output.jpg", "wb");
if (outfile == NULL) {
printf("Error opening output file");
return;
}
jpeg_stdio_dest(&cinfo, outfile);
/* Set the image parameters */
cinfo.image_width = width;
cinfo.image_height = height;
cinfo.input_components = 3;
cinfo.in_color_space = JCS_RGB;
/* Set the compression parameters */
jpeg_set_defaults(&cinfo);
jpeg_set_quality(&cinfo, quality, TRUE);
/* Start the compressor */
jpeg_start_compress(&cinfo, TRUE);
/* Write the image data */
unsigned char *row_pointer;
while (cinfo.next_scanline < cinfo.image_height) {
row_pointer = &image[cinfo.next_scanline * width * 3];
jpeg_write_scanlines(&cinfo, &row_pointer, 1);
}
/* Finish compression and release resources */
jpeg_finish_compress(&cinfo);
jpeg_destroy_compress(&cinfo);
fclose(outfile);
```
注意,以上代码仅供参考,具体实现可能需要根据具体的应用场景进行调整。
阅读全文
相关推荐
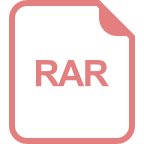
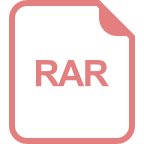
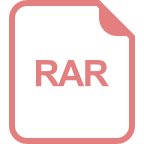
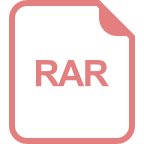
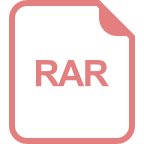
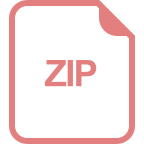
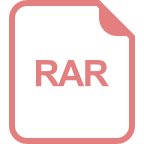
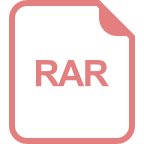
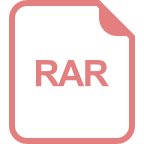
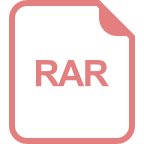
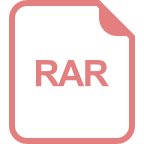
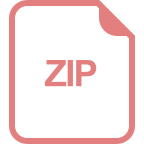