canny算子边缘检测原理,步骤和代码实现
时间: 2023-10-03 17:03:07 浏览: 111
Canny算子是一种常用的边缘检测方法,它的基本原理是利用图像中像素点灰度值的变化来检测边缘。其步骤如下:
1. 高斯滤波:对原始图像进行高斯滤波,平滑图像降低噪声干扰。
2. 计算梯度:利用Sobel算子计算图像的梯度值,求出每个像素点的梯度幅值和方向。
3. 非极大值抑制:对梯度图像进行非极大值抑制,保留梯度方向上的局部最大值,抑制其他值,从而使边缘更加细化。
4. 双阈值检测:根据设定的高低阈值,将梯度幅值分成强边缘、弱边缘和非边缘三个部分。只有强边缘和与之相邻的弱边缘才被认为是真正的边缘,其他部分被认为是噪声。
5. 连通分析:根据强边缘和弱边缘的连通性,将它们分成若干条边缘线段。
Canny算子的代码实现如下:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg', 0)
# 高斯滤波
img_blur = cv2.GaussianBlur(img, (3, 3), 0)
# 计算梯度
sobelx = cv2.Sobel(img_blur, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(img_blur, cv2.CV_64F, 0, 1, ksize=3)
grad_mag = np.sqrt(sobelx ** 2 + sobely ** 2)
grad_dir = np.arctan2(sobely, sobelx) * 180 / np.pi
# 非极大值抑制
grad_mag_max = cv2.dilate(grad_mag, np.ones((3, 3)))
grad_mag_nms = np.zeros(grad_mag.shape)
grad_mag_nms[(grad_mag == grad_mag_max) & (grad_mag > 5)] = grad_mag[(grad_mag == grad_mag_max) & (grad_mag > 5)]
# 双阈值检测
low_threshold = 40
high_threshold = 80
strong_edges = (grad_mag_nms > high_threshold).astype(np.uint8)
weak_edges = ((grad_mag_nms >= low_threshold) & (grad_mag_nms <= high_threshold)).astype(np.uint8)
# 连通分析
_, strong_edges = cv2.threshold(strong_edges, 0, 255, cv2.THRESH_BINARY)
_, labels = cv2.connectedComponents(strong_edges)
label_values = np.arange(1, labels.max() + 1)
edge_points = []
for label in label_values:
edge_points.append(np.column_stack(np.where(labels == label)))
edge_lines = []
for edge_point in edge_points:
if edge_point.shape[0] < 5:
continue
edge_line = cv2.fitLine(edge_point, cv2.DIST_L2, 0, 0.01, 0.01)
edge_lines.append(edge_line)
# 绘制边缘
for line in edge_lines:
vx, vy, x0, y0 = line
x1 = int((img.shape[0] - y0) * vx / vy + x0)
x2 = int(-y0 * vx / vy + x0)
cv2.line(img, (x1, img.shape[0]), (x2, 0), (0, 0, 255), 1)
cv2.imshow('edge detection', img)
cv2.waitKey()
cv2.destroyAllWindows()
```
阅读全文
相关推荐
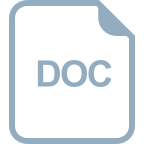
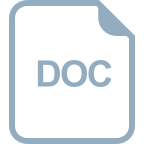
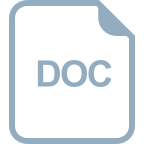
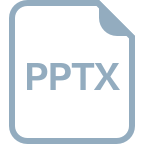
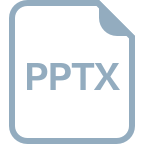
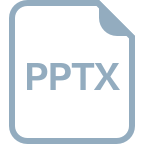
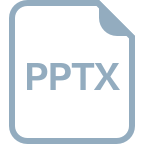
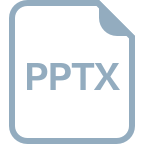


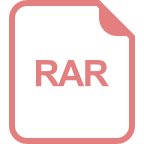
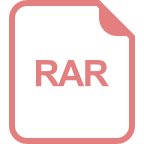
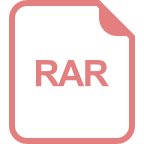
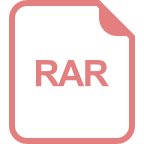
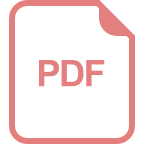
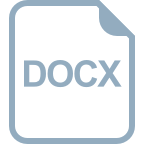
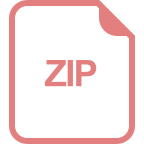
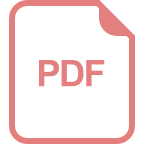
