不使用prune函数,对已经训练好的超分辨率模型剪枝代码
时间: 2024-03-01 07:51:52 浏览: 168
对已经训练好的超分辨率模型进行剪枝,可以使用以下步骤:
1. 加载已经训练好的模型。
```python
import torch
from model import SuperResolutionModel
model = SuperResolutionModel() # 实例化模型
# 加载已经训练好的模型参数
model.load_state_dict(torch.load('model.pth'))
```
2. 计算每个卷积层的权重。
```python
weights = []
for name, param in model.named_parameters():
if 'conv' in name and 'weight' in name:
weights.append(param)
```
3. 使用 KMeans 对权重进行聚类,并找到需要保留的中心点。
```python
from sklearn.cluster import KMeans
n_clusters = 16 # 聚类数量
kmeans = KMeans(n_clusters=n_clusters, n_jobs=-1)
kmeans.fit(torch.cat(weights).detach().cpu().numpy())
centers = []
for i in range(n_clusters):
cluster_center = torch.from_numpy(kmeans.cluster_centers_[i]).float().cuda()
centers.append(cluster_center)
```
4. 对模型进行剪枝,并更新卷积层的权重。
```python
idx = 0
for name, module in model.named_modules():
if isinstance(module, torch.nn.Conv2d):
weight = module.weight.data
bias = module.bias.data if module.bias is not None else None
n_filters = weight.shape[0]
# 计算每个卷积核的 L2 范数,并根据聚类结果找到需要保留的卷积核
norms = torch.norm(weight.view(n_filters, -1), 2, 1)
indices = torch.argmin(torch.cdist(norms.reshape(-1, 1), torch.cat([c.view(1, -1) for c in centers])), dim=1)
# 剪枝,保留需要的卷积核
weight = torch.index_select(weight, 0, indices)
if bias is not None:
bias = torch.index_select(bias, 0, indices)
# 更新卷积层的权重
module.weight.data = weight
if bias is not None:
module.bias.data = bias
idx += 1
# 保存剪枝后的模型
torch.save(model.state_dict(), 'pruned_model.pth')
```
这里采用的是 KMeans 算法对权重进行聚类,找到需要保留的中心点。在实际使用中,可以根据实际情况选择其他聚类算法或剪枝策略。
阅读全文
相关推荐
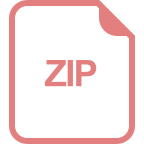
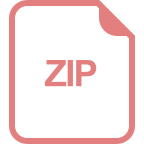
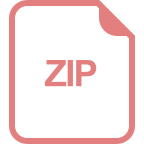





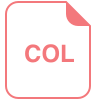
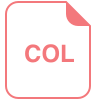
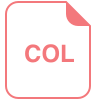
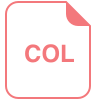
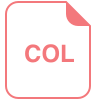
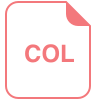
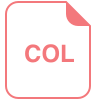
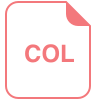
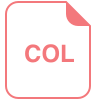
