多重继承 DateTime类 现有时间Date类和时间Time类,要求从两者中派生出DateTime类,具体的: Date类包含成员数据:年、月、日,包含成员函数:1)构造函数Date(int y, int m, int d) 2)void SetDate(int y, int m, int d) 设置时间,这两个函数均要求判断日期的正确性 3)void print(),以"2014/12/9",即YYYY/MM/DD的形式输出数据 Time类包含成员数据:时、分、秒,包含成员函数:1)构造函数Time(int h, int m, int s) 2)void SetTime(int y, int m, int s) 设置时间,这两个函数均要求判断时间的正确性 3)void print(),以"18:21:0",即以hh:mm:ss的形式输出数据 DateTime类包含1)构造函数DateTime(ye, mo, da, ho, mi, se) ,2)显示日期时间 void print() 以“2014/12/9 18:21:00”的形式输出时间
时间: 2024-03-12 22:49:25 浏览: 81
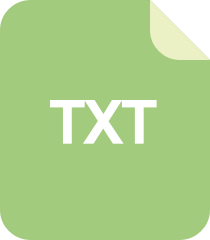
在C++多继承体系当中,在派生类中可以重写不同基类中的虚函数。下面就是一个例子:

好的,根据您的要求,可以定义如下的三个类:
```c++
#include <iostream>
using namespace std;
class Date {
public:
Date(int y, int m, int d) {
SetDate(y, m, d);
}
void SetDate(int y, int m, int d) {
year = y;
month = m;
day = d;
// 判断日期的正确性
if (month < 1 || month > 12) {
cout << "Error: Invalid month!" << endl;
exit(1);
}
if (day < 1 || day > GetMaxDay()) {
cout << "Error: Invalid day!" << endl;
exit(1);
}
}
void print() {
cout << year << "/" << month << "/" << day;
}
protected:
int year, month, day;
private:
bool IsLeapYear() {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
int GetMaxDay() {
static const int max_day[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (month == 2 && IsLeapYear()) {
return 29;
} else {
return max_day[month];
}
}
};
class Time {
public:
Time(int h, int m, int s) {
SetTime(h, m, s);
}
void SetTime(int h, int m, int s) {
hour = h;
minute = m;
second = s;
// 判断时间的正确性
if (hour < 0 || hour > 23) {
cout << "Error: Invalid hour!" << endl;
exit(1);
}
if (minute < 0 || minute > 59) {
cout << "Error: Invalid minute!" << endl;
exit(1);
}
if (second < 0 || second > 59) {
cout << "Error: Invalid second!" << endl;
exit(1);
}
}
void print() {
cout << hour << ":" << minute << ":" << second;
}
protected:
int hour, minute, second;
};
class DateTime : public Date, public Time {
public:
DateTime(int ye, int mo, int da, int ho, int mi, int se) :
Date(ye, mo, da), Time(ho, mi, se) {}
void print() {
Date::print();
cout << " ";
Time::print();
}
};
```
这个实现中,Date类和Time类都提供了构造函数和print()函数,其中Date类还提供了SetDate()函数,用于设置日期,Time类还提供了SetTime()函数,用于设置时间。DateTime类继承了Date类和Time类,并提供了自己的构造函数和print()函数,其中构造函数使用了初始化列表来初始化Date和Time的成员。在DateTime的print()函数中,我们分别调用了Date::print()和Time::print()函数,并在它们之间输出一个空格,从而实现了以“2014/12/9 18:21:00”的形式输出时间的功能。
阅读全文
相关推荐
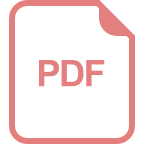
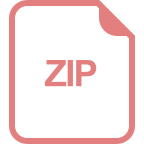
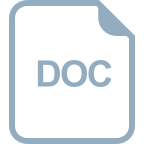




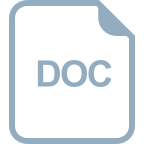
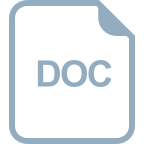
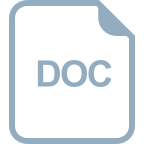
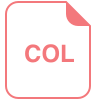
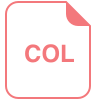
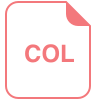
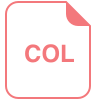
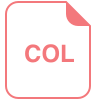
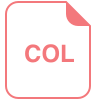
