Devc++电影院管理系统代码
时间: 2023-08-17 11:04:08 浏览: 46
这里提供一个简单的Dev-C++电影院管理系统的代码示例,包括了电影信息的录入、查询和场次的安排等功能。具体实现还需要根据实际需求进行完善和修改。
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 电影类
class Movie {
public:
Movie(string name, string director, string actor, string date, float price) {
this->name = name;
this->director = director;
this->actor = actor;
this->date = date;
this->price = price;
}
string getName() {
return name;
}
string getDirector() {
return director;
}
string getActor() {
return actor;
}
string getDate() {
return date;
}
float getPrice() {
return price;
}
private:
string name; // 电影名称
string director; // 导演
string actor; // 主演
string date; // 上映日期
float price; // 票价
};
// 场次类
class ShowTime {
public:
ShowTime(Movie* movie, string time, int room, int seats) {
this->movie = movie;
this->time = time;
this->room = room;
this->seats = seats;
this->soldSeats = 0;
}
Movie* getMovie() {
return movie;
}
string getTime() {
return time;
}
int getRoom() {
return room;
}
int getSeats() {
return seats;
}
int getSoldSeats() {
return soldSeats;
}
void sellTicket(int num) {
soldSeats += num;
}
private:
Movie* movie; // 电影信息
string time; // 上映时间
int room; // 放映厅
int seats; // 座位数
int soldSeats; // 已售座位数
};
// 电影院类
class Cinema {
public:
void addMovie(Movie* movie) {
movies.push_back(movie);
}
void addShowTime(ShowTime* showTime) {
showTimes.push_back(showTime);
}
void printMovies() {
cout << "电影列表:" << endl;
for (int i = 0; i < movies.size(); i++) {
Movie* movie = movies[i];
cout << "[" << i + 1 << "] " << movie->getName() << endl;
}
}
void queryMovies(string name) {
cout << "查询结果:" << endl;
for (int i = 0; i < movies.size(); i++) {
Movie* movie = movies[i];
if (movie->getName() == name) {
cout << "[" << i + 1 << "] " << movie->getName() << " " << movie->getDirector() << " " << movie->getActor() << " " << movie->getDate() << " " << movie->getPrice() << endl;
}
}
}
void arrangeShowTime(int movieIndex, string time, int room, int seats) {
Movie* movie = movies[movieIndex - 1];
ShowTime* showTime = new ShowTime(movie, time, room, seats);
showTimes.push_back(showTime);
cout << "场次已安排:" << movie->getName() << " " << time << " " << room << " " << seats << endl;
}
void sellTicket(int showTimeIndex, int num) {
ShowTime* showTime = showTimes[showTimeIndex - 1];
if (showTime->getSeats() - showTime->getSoldSeats() < num) {
cout << "座位不足,无法购买" << endl;
return;
}
float price = showTime->getMovie()->getPrice();
float total = price * num;
cout << "购票成功:" << showTime->getMovie()->getName() << " " << showTime->getTime() << " " << num << "张 " << total << "元" << endl;
showTime->sellTicket(num);
}
void printShowTimes() {
cout << "场次列表:" << endl;
for (int i = 0; i < showTimes.size(); i++) {
ShowTime* showTime = showTimes[i];
cout << "[" << i + 1 << "] " << showTime->getMovie()->getName() << " " << showTime->getTime() << " " << showTime->getRoom() << " " << showTime->getSeats() << " " << showTime->getSoldSeats() << endl;
}
}
private:
vector<Movie*> movies; // 电影列表
vector<ShowTime*> showTimes; // 场次列表
};
int main() {
Cinema cinema;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 录入电影信息" << endl;
cout << "2. 查询电影信息" << endl;
cout << "3. 安排场次" << endl;
cout << "4. 售票" << endl;
cout << "5. 查看场次" << endl;
cout << "0. 退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
cout << "请输入电影信息:" << endl;
string name, director, actor, date;
float price;
cin >> name >> director >> actor >> date >> price;
Movie* movie = new Movie(name, director, actor, date, price);
cinema.addMovie(movie);
cout << "电影信息已录入:" << name << " " << director << " " << actor << " " << date << " " << price << endl;
break;
}
case 2: {
cout << "请输入电影名称:" << endl;
string name;
cin >> name;
cinema.queryMovies(name);
break;
}
case 3: {
cinema.printMovies();
cout << "请选择电影:" << endl;
int movieIndex;
cin >> movieIndex;
cout << "请输入场次信息:" << endl;
string time;
int room, seats;
cin >> time >> room >> seats;
cinema.arrangeShowTime(movieIndex, time, room, seats);
break;
}
case 4: {
cinema.printShowTimes();
cout << "请选择场次:" << endl;
int showTimeIndex;
cin >> showTimeIndex;
cout << "请输入购票数量:" << endl;
int num;
cin >> num;
cinema.sellTicket(showTimeIndex, num);
break;
}
case 5: {
cinema.printShowTimes();
break;
}
case 0: {
return 0;
}
default: {
cout << "无效操作,请重新输入!" << endl;
break;
}
}
}
return 0;
}
```
相关推荐
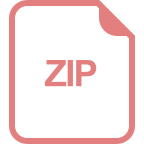















