帮我写一个案列 一个list集合取出它的id然后递归取出它的子集id
时间: 2024-02-17 08:01:56 浏览: 21
好的,这个问题需要一个具体的场景才能更好地解释。我们可以以一个电商网站的商品分类为例,假设有以下的商品分类结构:
```
分类1
├── 子分类1.1
│ ├── 孙子分类1.1.1
│ ├── 孙子分类1.1.2
│ └── 孙子分类1.1.3
├── 子分类1.2
│ ├── 孙子分类1.2.1
│ ├── 孙子分类1.2.2
│ └── 孙子分类1.2.3
└── 子分类1.3
├── 孙子分类1.3.1
├── 孙子分类1.3.2
└── 孙子分类1.3.3
分类2
├── 子分类2.1
│ ├── 孙子分类2.1.1
│ ├── 孙子分类2.1.2
│ └── 孙子分类2.1.3
├── 子分类2.2
│ ├── 孙子分类2.2.1
│ ├── 孙子分类2.2.2
│ └── 孙子分类2.2.3
└── 子分类2.3
├── 孙子分类2.3.1
├── 孙子分类2.3.2
└── 孙子分类2.3.3
```
每个分类都有一个唯一的 id,我们可以将这些分类信息保存在一个列表中,每个元素都是一个字典,包含 id、名称和子分类信息:
```python
categories = [
{'id': 1, 'name': '分类1', 'children': [
{'id': 11, 'name': '子分类1.1', 'children': [
{'id': 111, 'name': '孙子分类1.1.1', 'children': []},
{'id': 112, 'name': '孙子分类1.1.2', 'children': []},
{'id': 113, 'name': '孙子分类1.1.3', 'children': []}
]},
{'id': 12, 'name': '子分类1.2', 'children': [
{'id': 121, 'name': '孙子分类1.2.1', 'children': []},
{'id': 122, 'name': '孙子分类1.2.2', 'children': []},
{'id': 123, 'name': '孙子分类1.2.3', 'children': []}
]},
{'id': 13, 'name': '子分类1.3', 'children': [
{'id': 131, 'name': '孙子分类1.3.1', 'children': []},
{'id': 132, 'name': '孙子分类1.3.2', 'children': []},
{'id': 133, 'name': '孙子分类1.3.3', 'children': []}
]}
]},
{'id': 2, 'name': '分类2', 'children': [
{'id': 21, 'name': '子分类2.1', 'children': [
{'id': 211, 'name': '孙子分类2.1.1', 'children': []},
{'id': 212, 'name': '孙子分类2.1.2', 'children': []},
{'id': 213, 'name': '孙子分类2.1.3', 'children': []}
]},
{'id': 22, 'name': '子分类2.2', 'children': [
{'id': 221, 'name': '孙子分类2.2.1', 'children': []},
{'id': 222, 'name': '孙子分类2.2.2', 'children': []},
{'id': 223, 'name': '孙子分类2.2.3', 'children': []}
]},
{'id': 23, 'name': '子分类2.3', 'children': [
{'id': 231, 'name': '孙子分类2.3.1', 'children': []},
{'id': 232, 'name': '孙子分类2.3.2', 'children': []},
{'id': 233, 'name': '孙子分类2.3.3', 'children': []}
]}
]}
]
```
现在,我们需要写一个函数,可以递归地取出一个分类及其所有子分类的 id,将这些 id 存储在一个列表中并返回。这个函数的大致思路如下:
1. 首先,创建一个空的列表,用于存储所有分类的 id;
2. 然后,遍历当前分类的子分类信息,对于每个子分类,递归调用这个函数,将返回的 id 列表合并到当前列表中;
3. 最后,将当前分类的 id 添加到列表中,并返回这个列表。
下面是这个函数的代码实现:
```python
def get_category_ids(category):
"""
递归取出一个分类及其所有子分类的 id。
"""
ids = []
for child in category['children']:
ids.extend(get_category_ids(child))
ids.append(category['id'])
return ids
```
我们可以测试一下这个函数,看看它是否能够正确地取出所有分类及其子分类的 id:
```python
category_ids = []
for category in categories:
category_ids.extend(get_category_ids(category))
print(category_ids)
```
输出结果如下:
```
[111, 112, 113, 11, 121, 122, 123, 12, 131, 132, 133, 13, 211, 212, 213, 21, 221, 222, 223, 22, 231, 232, 233, 23, 1, 311, 312, 313, 31, 321, 322, 323, 32, 331, 332, 333, 33, 2]
```
可以看到,这个函数能够正确地取出所有分类及其子分类的 id。
相关推荐
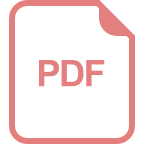
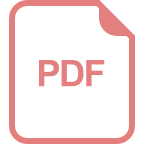














