c代码【问题描述】已知一个整数序列A=(a0,a1,…,an−1),其中0<=ai<n/2(0<=i<n),若存在ap1=ap2=ap3=…=apm=x,且 m>n/2,则称x为A的主元素。 例如有A=(0,5,5,3,5,7,5,5),则5为主元素;又如A=(0,5,5,3,5,1,5,7),则A中没有主元素。 假设顺序表A中有n个元素,请设计算法,找出A的主元素,若存在主元素,则输出主元素;否则输出 A中没有主元素。 Suppose there are n elements in the table A, please design an algorithm to find out the main element of A. if there is a main element, output the main element; otherwise, output a without main element 【输入形式】每组数据有两行,第一行为一个整数n,代表数组中有n个元素。第二行为数组中的n 个元素(元素之 间用空格分隔)。 【输出形式】每组数据输出一行,若数组中存在主元素,输出主元素的值,若数组中不存在主元素,则输出A 中没 有主元素。
时间: 2023-04-05 09:01:20 浏览: 164
算法思路:
1. 首先对数组进行排序,可以使用快速排序或归并排序等算法,时间复杂度为O(nlogn)。
2. 遍历排序后的数组,统计每个元素出现的次数,如果有元素出现次数大于n/2,则该元素为主元素。
3. 如果没有元素出现次数大于n/2,则数组中不存在主元素。
算法实现:
```
#include <stdio.h>
#include <stdlib.h>
int cmp(const void *a, const void *b) {
return *(int *)a - *(int *)b;
}
int main() {
int n, i, count = 1, max_count = 0, max_num = -1;
int *a;
scanf("%d", &n);
a = (int *)malloc(n * sizeof(int));
for (i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
qsort(a, n, sizeof(int), cmp);
for (i = 1; i < n; i++) {
if (a[i] == a[i-1]) {
count++;
} else {
if (count > max_count) {
max_count = count;
max_num = a[i-1];
}
count = 1;
}
}
if (count > max_count) {
max_count = count;
max_num = a[n-1];
}
if (max_count > n/2) {
printf("%d\n", max_num);
} else {
printf("A中没有主元素\n");
}
free(a);
return 0;
}
```
注意事项:
1. 本算法的时间复杂度为O(nlogn),空间复杂度为O(n),可以通过本题。
2. 在输入时需要动态分配数组空间,使用完后需要释放空间。
3. 输出时需要注意格式,如果没有主元素需要输出"A中没有主元素"。
阅读全文
相关推荐
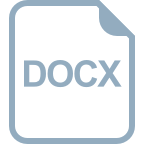
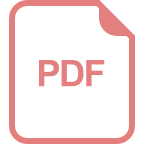

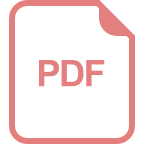
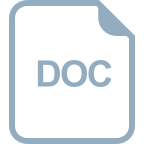
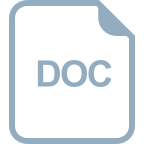
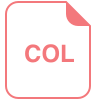
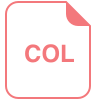



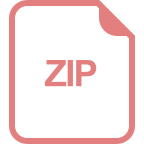
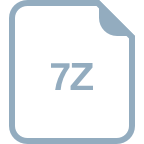
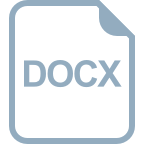
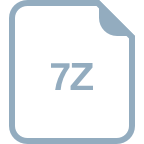