窗口排队管理系统代码
时间: 2023-08-06 13:24:31 浏览: 51
这里提供一个简单的窗口排队管理系统的代码示例,供参考:
```python
# 定义窗口类
class Window:
def __init__(self, id, business_type, worker_id):
self.id = id
self.business_type = business_type
self.queue = []
self.worker_id = worker_id
self.status = '空闲'
def __str__(self):
return f'窗口{self.id} ({self.business_type})'
# 定义窗口管理类
class WindowManager:
def __init__(self):
self.windows = []
def add_window(self, window):
self.windows.append(window)
def remove_window(self, window):
if window.status != '空闲':
raise ValueError('存在排队的顾客,无法删除该窗口')
self.windows.remove(window)
def get_windows(self):
return self.windows
def get_window_by_id(self, id):
for window in self.windows:
if window.id == id:
return window
return None
def set_window_status(self, window, status):
if status not in ['空闲', '忙碌', '暂停']:
raise ValueError('无效的窗口状态')
window.status = status
def save_windows(self, filename):
with open(filename, 'w') as f:
for window in self.windows:
f.write(f'{window.id},{window.business_type},{window.worker_id},{window.status}\n')
def load_windows(self, filename):
with open(filename, 'r') as f:
lines = f.readlines()
for line in lines:
values = line.strip().split(',')
id = int(values[0])
business_type = values[1]
worker_id = int(values[2])
status = values[3]
window = Window(id, business_type, worker_id)
self.set_window_status(window, status)
self.add_window(window)
# 定义顾客类
class Customer:
def __init__(self, id, business_type):
self.id = id
self.business_type = business_type
# 定义营业厅类
class Hall:
def __init__(self, window_manager, max_queue_size):
self.window_manager = window_manager
self.max_queue_size = max_queue_size
self.customers = []
def add_customer(self, customer):
if len(self.customers) >= self.max_queue_size:
raise ValueError('排队人数已达上限')
self.customers.append(customer)
def remove_customer(self, customer):
self.customers.remove(customer)
def get_customers(self):
return self.customers
def assign_window(self, customer):
assigned = False
for window in self.window_manager.get_windows():
if window.business_type == customer.business_type and window.status == '空闲':
window.queue.append(customer)
self.window_manager.set_window_status(window, '忙碌')
assigned = True
break
if not assigned:
shortest_queue = None
for window in self.window_manager.get_windows():
if window.business_type == customer.business_type and window.status == '忙碌':
continue
if shortest_queue is None or len(window.queue) < len(shortest_queue.queue):
shortest_queue = window
shortest_queue.queue.append(customer)
def serve_customer(self):
earliest_customer = None
earliest_window = None
for window in self.window_manager.get_windows():
if window.status != '忙碌':
continue
if len(window.queue) == 0:
self.window_manager.set_window_status(window, '空闲')
continue
customer = window.queue[0]
if earliest_customer is None or customer.id < earliest_customer.id:
earliest_customer = customer
earliest_window = window
if earliest_customer is not None:
earliest_window.queue.remove(earliest_customer)
self.remove_customer(earliest_customer)
self.window_manager.set_window_status(earliest_window, '空闲')
return earliest_window, earliest_customer
return None
def save_customers(self, filename):
with open(filename, 'w') as f:
for customer in self.customers:
f.write(f'{customer.id},{customer.business_type}\n')
def load_customers(self, filename):
with open(filename, 'r') as f:
lines = f.readlines()
for line in lines:
values = line.strip().split(',')
id = int(values[0])
business_type = values[1]
customer = Customer(id, business_type)
self.add_customer(customer)
# 测试代码
if __name__ == '__main__':
# 创建窗口管理器
window_manager = WindowManager()
# 添加窗口
window1 = Window(1, '存款', 101)
window_manager.add_window(window1)
window2 = Window(2, '取款', 102)
window_manager.add_window(window2)
window3 = Window(3, '转账', 103)
window_manager.add_window(window3)
# 保存窗口信息到文件
window_manager.save_windows('windows.txt')
# 创建营业厅
hall = Hall(window_manager, 5)
# 添加顾客
customer1 = Customer(1, '存款')
hall.add_customer(customer1)
customer2 = Customer(2, '取款')
hall.add_customer(customer2)
customer3 = Customer(3, '转账')
hall.add_customer(customer3)
# 保存顾客信息到文件
hall.save_customers('customers.txt')
# 分配窗口
hall.assign_window(customer1)
hall.assign_window(customer2)
hall.assign_window(customer3)
# 办理业务
window, customer = hall.serve_customer()
print(f'{window} 办理 {customer} 的业务')
window, customer = hall.serve_customer()
print(f'{window} 办理 {customer} 的业务')
window, customer = hall.serve_customer()
print(f'{window} 办理 {customer} 的业务')
# 加载窗口和顾客信息
window_manager.load_windows('windows.txt')
hall.load_customers('customers.txt')
# 查看窗口状态
for window in window_manager.get_windows():
print(f'{window}: {len(window.queue)} 人在排队')
```
注:以上示例代码中的实现只是一种可能的实现方式,具体实现细节和业务逻辑可以根据实际情况进行调整和优化。
相关推荐
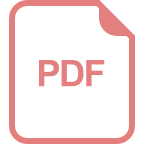













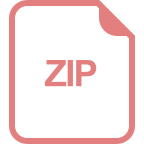
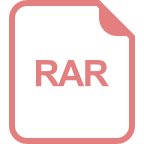