unity如何获取buildsetting里面的scene
时间: 2024-05-04 19:16:45 浏览: 13
可以使用以下代码来获取 BuildSettings 中所有的场景路径:
```csharp
using UnityEngine.SceneManagement;
string[] scenePaths = new string[SceneManager.sceneCountInBuildSettings];
for (int i = 0; i < scenePaths.Length; i++)
{
scenePaths[i] = SceneUtility.GetScenePathByBuildIndex(i);
}
```
这将返回一个字符串数组,其中包含 BuildSettings 中所有场景的路径。
相关问题
unity buildsetting
Unity Build Setting是Unity中的一个功能,用于配置和管理项目的构建设置。通过Build Setting,用户可以选择构建平台、添加场景、设置构建选项等。这个功能非常重要,因为它直接影响到项目的构建和发布。
unity build setting中clean build和build的区别
在Unity的Build Settings中,Clean Build和Build两个选项的区别在于:
1. Clean Build会在生成新的Build之前清除所有的中间文件、缓存和已有的Build文件。这意味着您需要重新编译整个项目,并且生成的Build文件将是全新的。
2. Build选项只会编译最新更改的代码和资源,然后将其添加到现有的Build文件中。这意味着Build速度会更快,但是可能会出现一些已解决的错误或无用的文件被包含在内的情况。
因此,如果您需要生成全新的Build文件并确保所有中间文件和缓存都已清除,请选择Clean Build。如果您只想生成最新更改的代码并快速生成Build文件,请选择Build。
相关推荐
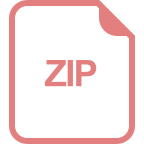
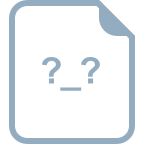












