c语言 调用fork命令 在子进程中打印子进程pid
时间: 2024-05-15 14:17:19 浏览: 18
好的,这是一个技术问题,我可以回答。在C语言中,调用fork命令可以创建一个子进程。在子进程中可以使用getpid函数获取子进程的进程ID,然后再使用printf函数打印该ID号即可。以下是一个示例代码:
```c
#include <stdio.h>
#include <unistd.h>
int main() {
int pid = fork();
if (pid == 0) {
printf("子进程的进程ID是:%d\n", getpid());
}
return 0;
}
```
相关问题
linux在C语言中如何用kill向子进程发送signal
在Linux中,可以使用kill函数向另一个进程发送信号,其函数原型如下:
```c
#include <signal.h>
int kill(pid_t pid, int sig);
```
其中,pid参数表示要发送信号的进程的ID,sig参数表示要发送的信号类型。
如果要向子进程发送信号,可以在父进程中使用fork函数创建子进程,并在子进程中使用kill函数发送信号。例如:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
void child_handler(int sig) {
printf("Child process received signal %d\n", sig);
}
int main() {
pid_t pid = fork();
if (pid < 0) {
printf("Failed to fork.\n");
return 1;
} else if (pid == 0) {
// Child process
signal(SIGINT, child_handler); // Set signal handler
while (1) {
sleep(1); // Wait for signal
}
} else {
// Parent process
sleep(2); // Wait for child to initialize
kill(pid, SIGINT); // Send signal to child
}
return 0;
}
```
在上面的示例中,父进程使用kill函数向子进程发送SIGINT信号,子进程在接收到信号后会调用child_handler函数进行处理。注意,在子进程中需要使用signal函数设置信号处理函数。
用C语言编程实现父进程fork子进程的功能。 调用fork命令,并在子进程中打印子进程pid; 在子进程中sleep 10秒; i. 若父进程不愿等待这10秒而直接退出,编写程序直接打印父进程id,程序名myshell_nowait,观察进程的僵尸状态; ii. 若父进程必须等待子进程结束,打印父进程id并调用waitpid打印子进程id, 程序名myshell_wait;
下面是用C语言编写的两个程序:myshell_nowait和myshell_wait,分别实现了父进程不等待子进程和父进程等待子进程的功能。
myshell_nowait程序:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid;
pid = fork();
if (pid < 0) {
printf("fork error\n");
return -1;
} else if (pid == 0) {
printf("child pid: %d\n", getpid());
sleep(10);
printf("child process exit\n");
} else {
printf("parent pid: %d\n", getpid());
printf("parent process exit\n");
}
return 0;
}
```
myshell_wait程序:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid;
int status;
pid = fork();
if (pid < 0) {
printf("fork error\n");
return -1;
} else if (pid == 0) {
printf("child pid: %d\n", getpid());
sleep(10);
printf("child process exit\n");
} else {
printf("parent pid: %d\n", getpid());
waitpid(pid, &status, 0);
printf("child pid: %d exit\n", pid);
printf("parent process exit\n");
}
return 0;
}
```
两个程序的区别在于myshell_wait使用了waitpid函数等待子进程结束并获取子进程的状态,而myshell_nowait直接退出了父进程。执行myshell_wait程序时,可以看到父进程会等待子进程结束并打印出子进程的pid,而执行myshell_nowait程序时,如果不等待子进程,子进程将变成僵尸进程。
相关推荐
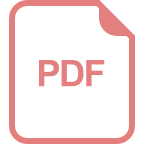
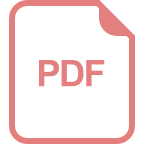
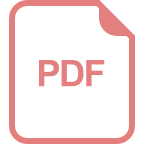












