帮我用C语言实现一个程序,该程序模拟进程的先来先服务算法,每个PCB包括随机生成的状态、运行时间、优先级,使用等待队列和就绪队列
时间: 2023-06-07 17:11:21 浏览: 48
好的,根据您的要求,我可以帮您用C语言实现进程的先来先服务算法。以下是代码实现:
```
#include <stdio.h>
#include <stdlib.h>
struct ProcessControlBlock {
int pid; // 进程ID
int status; // 进程状态,0表示就绪,1表示运行,2表示等待
int totalTime; // 进程需要执行的总时间
int executedTime; // 已执行的时间
int priority; // 进程优先级
struct ProcessControlBlock* next; // 进程控制块指针
};
// 初始化PCB队列
void init(struct ProcessControlBlock** queue) {
*queue = NULL;
}
// 添加PCB到队列
void enqueue(struct ProcessControlBlock** queue, struct ProcessControlBlock* process) {
if (*queue == NULL) {
*queue = process;
(*queue) -> next = NULL;
} else if (process -> priority < (*queue) -> priority) {
process -> next = *queue;
*queue = process;
} else {
struct ProcessControlBlock* current = *queue;
while (current -> next != NULL &&
current -> next -> priority <= process -> priority) {
current = current -> next;
}
process -> next = current -> next;
current -> next = process;
}
}
// 从队列中移除PCB
struct ProcessControlBlock* dequeue(struct ProcessControlBlock** queue) {
if (*queue == NULL) {
return NULL;
}
struct ProcessControlBlock* temp = *queue;
*queue = (*queue) -> next;
return temp;
}
// 进程调度
void schedule(struct ProcessControlBlock** readyQueue, struct ProcessControlBlock** waitQueue) {
if (*readyQueue == NULL && *waitQueue == NULL) {
printf("PCB队列为空!\n");
return;
}
if (*readyQueue != NULL) {
struct ProcessControlBlock* running = dequeue(readyQueue);
running -> status = 1;
running -> executedTime++;
running -> totalTime--;
printf("当前运行的进程:%d,已执行的时间:%d,优先级:%d \n",
running -> pid, running -> executedTime, running -> priority);
if (running -> totalTime == 0) {
printf("进程 %d 执行完毕!\n", running -> pid);
free(running);
} else {
running -> status = 0;
enqueue(readyQueue, running);
}
} else {
struct ProcessControlBlock* waiting = dequeue(waitQueue);
waiting -> status = 0;
enqueue(readyQueue, waiting);
}
}
int main() {
struct ProcessControlBlock *readyQueue, *waitQueue, *proc;
int pid = 0;
int i;
init(&readyQueue);
init(&waitQueue);
// 生成5个PCB,每个随机分配状态、运行时间和优先级
for (i = 0; i < 5; i++) {
proc = (struct ProcessControlBlock*) malloc(sizeof(struct ProcessControlBlock));
proc -> pid = pid++;
proc -> status = rand() % 3;
proc -> totalTime = rand() % 11 + 5;
proc -> executedTime = 0;
proc -> priority = rand() % 10;
if (proc -> status == 0) {
enqueue(&readyQueue, proc);
} else {
enqueue(&waitQueue, proc);
}
}
// 进程调度
while (readyQueue != NULL || waitQueue != NULL) {
schedule(&readyQueue, &waitQueue);
}
return 0;
}
```
该程序模拟进程的先来先服务算法,每个PCB包括随机生成的状态、运行时间、优先级,使用等待队列和就绪队列,当就绪队列为空时,调度等待队列中的进程,直到所有进程执行完毕。
相关推荐
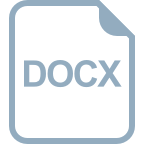








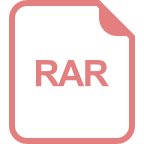
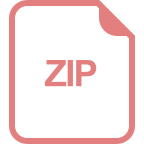
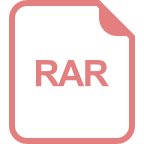