java输入某年某月某日,并判断这一天是这一年的第几天
时间: 2023-05-31 08:20:17 浏览: 233
### 回答1:
首先,需要根据输入的年、月、日生成一个日期对象。可以使用Java自带的Calendar工具类来实现。
然后,需要判断输入的年份是否是闰年。闰年的判定方法是:能被4整除但不能被100整除,或者能被400整除。
接下来,可以使用循环来计算出从该年的1月1日到输入的这一天,一共经过了多少天。具体方法是:先将天数加上输入的日数,然后根据输入的月份循环,每次将天数加上该月份的天数。
最后输出计算得到的天数即可。
代码如下:
```
import java.util.Calendar;
public class DayOfYear {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入年份:");
int year = scanner.nextInt();
System.out.println("请输入月份:");
int month = scanner.nextInt();
System.out.println("请输入日期:");
int day = scanner.nextInt();
Calendar calendar = Calendar.getInstance();
calendar.set(year, month - 1, day);
int days = day;
boolean isLeapYear = isLeapYear(year);
int[] monthDays = {31, isLeapYear ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
for (int i = 0; i < month - 1; i++) {
days += monthDays[i];
}
System.out.println(year + "年" + month + "月" + day + "日是这一年的第" + days + "天。");
}
// 判断是否是闰年
public static boolean isLeapYear(int year) {
return year % 4 == 0 && year % 100 != 0 || year % 400 == 0;
}
}
```
### 回答2:
Java是一款流行的编程语言,它可以实现输入某年某月某日的功能,并判断这一天是这一年的第几天。具体实现方法如下:
首先,我们需要使用Scanner类建立一个输入流,来读取用户输入的年、月、日。
接着,我们需要判断用户输入的年份是否为闰年。判断方法为:如果该年份能被4整除但不能被100整除,或能被400整除,则是闰年。
然后,我们需要计算该日期是该年的第几天。具体计算方法为:先将用户输入的月份前面所有月份的天数加起来,再加上用户输入的日数。如果该年为闰年,并且用户输入的月份大于2月,还需要再加上1天。
最后,我们将计算出来的结果输出即可。
以下是Java代码示例:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入年份:");
int year = input.nextInt();
System.out.print("请输入月份:");
int month = input.nextInt();
System.out.print("请输入日期:");
int day = input.nextInt();
if (isLeapYear(year) && month > 2) {
System.out.println("这一天是" + year + "年的第" + (getDaysOfMonth(month - 1, year) + day + 1) + "天");
} else {
System.out.println("这一天是" + year + "年的第" + (getDaysOfMonth(month - 1, year) + day) + "天");
}
}
// 判断是否为闰年
public static boolean isLeapYear(int year) {
return year % 4 == 0 && year % 100 != 0 || year % 400 == 0;
}
// 获取某个月份的天数
public static int getDaysOfMonth(int month, int year) {
int[] days = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
if (isLeapYear(year) && month == 1) {
return 29;
}
return days[month];
}
}
通过以上代码,我们就可以实现输入某年某月某日的功能,并判断这一天是这一年的第几天。
### 回答3:
首先,我们需要了解一下每个月有多少天,这可以通过一个数组来存储。在 Java 中,可以这样定义这个数组:
int[] days = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
其中,days[0] 表示一月有31天,days[1] 表示二月有28天(闰年的时候是29天),依此类推。
接下来,我们可以通过 Scanner 类来读取用户输入的年、月、日:
Scanner sc = new Scanner(System.in);
int year = sc.nextInt();
int month = sc.nextInt();
int day = sc.nextInt();
其中,nextInt() 方法用来读取一个整数。
接下来,需要计算这一天是这一年的第几天。我们可以先计算前面几个月的天数,再加上当前月的天数,得到这一年的总天数。具体实现可以参考下面的代码:
int totalDays = day;
for (int i = 0; i < month - 1; i++) {
totalDays += days[i];
}
if (month > 2 && isLeapYear(year)) {
totalDays++; // 闰年的二月多一天
}
System.out.println("这一天是这一年的第 " + totalDays + " 天");
其中,isLeapYear(year) 方法用来判断是否是闰年,这里不再赘述。
完整代码如下:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int[] days = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
Scanner sc = new Scanner(System.in);
int year = sc.nextInt();
int month = sc.nextInt();
int day = sc.nextInt();
int totalDays = day;
for (int i = 0; i < month - 1; i++) {
totalDays += days[i];
}
if (month > 2 && isLeapYear(year)) {
totalDays++; // 闰年的二月多一天
}
System.out.println("这一天是这一年的第 " + totalDays + " 天");
}
public static boolean isLeapYear(int year) {
return year % 4 == 0 && year % 100 != 0 || year % 400 == 0;
}
}
阅读全文
相关推荐
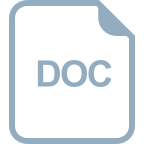
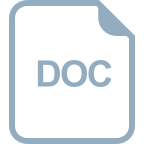
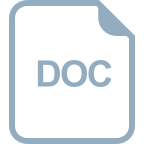
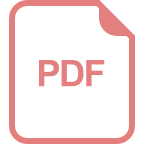




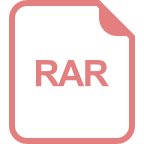









